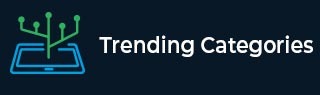
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Node.js – util.callbackify() Method
The util.callbackify() method takes an async function as a parameter (or a function with Promise) and returns a function with the callback style. The callback will hold the rejection reason as the first parameter (or null in case of Promise) and the resolved value as the second parameter.
Syntax
util.callbackify(function)
Parameters
- function − The input parameter for the async_function required for callback.
Example 1
Create a file "callbackify.js" and copy the following code snippet. After creating the file, use the command "node callbackify.js" to run this code.
// util.callbackify() demo example // Importing the util module const util = require('util'); // Defining a simple async function async function async_fn() { return 'Welcome to Turoials Point !!!'; } // Defining the util.callbackify() with async function const callbackFunction = util.callbackify(async_fn); // Consuming the callback callbackFunction((err, ret) => { if (err) throw err; console.log(ret); });
Output
C:\home
ode>> node callbackify.js Welcome to Turoials Point !!!
Example 2
The callback will throw an ‘uncaught exception’ event if any error or exception occurs while executing the function. If the callback is not handled, it will exit. Let’s try the callbackify() function with a Promise having null parameters.
// util.callbackify() demo example // Importing the util module const util = require('util'); // Defining a simple Promise function function async_fn() { return Promise.reject(null); } // Defining the callback function const callbackFunction = util.callbackify(async_fn); callbackFunction((err, ret) => { // The promise will be rejected with 'null', as it is wrapped // with an Error and the error message being stored in the 'reason' err && err.hasOwnProperty('reason') && err.reason === null; // true(since promise has error) console.log(err); });
Output
C:\home
ode>> node callbackify.js { Error [ERR_FALSY_VALUE_REJECTION]: Promise was rejected with falsy value at process._tickCallback (internal/process/next_tick.js:63:19) at Function.Module.runMain (internal/modules/cjs/loader.js:834:11) at startup (internal/bootstrap/node.js:283:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:623:3) reason: null }
Advertisements