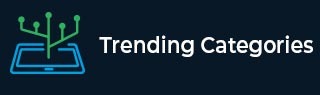
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Node.js – process.emitWarning() Method
The process.emitWarning() method can be used to emit custom or user-defined process warnings. This can be listened by adding a handler to the warning event.
Syntax
process.emitWarning(warning, [options])
Parameters
warning – This is the warning that will be emitted.
options –
type – This is the type of warning being emitted. Default ‘Warning’
code – This is a unique identifier for the warning that will be emitted.
ctor – This is an optional function used to limit the generated stack trace.
detail – This is the additional text to be included with the error.
Example 1
Create a file with the name "warning.js" and copy the following code snippet. After creating the file, use the command "node warning.js" to run this code.
console.log("Start ..."); // Interval set to keep the process running setInterval(() => { console.log("Running ...");}, 1000); setTimeout(() => { process.emitWarning('An Error occured!', { code: 'Custom_Warning', detail: 'Additional information about warning' }); }, 4000); // Start listening process.on('warning', (warning) => { // Print msg if there is a warning if (warning) { console.log(warning); process.exit(0); } });
Output
Start ... Running ... Running ... Running ... { [object Object]: An Error occured! at Timeout.setTimeout (/home/cg/root/8008764/main.js:8:12) at ontimeout (timers.js:386:14) at tryOnTimeout (timers.js:250:5) at Timer.listOnTimeout (timers.js:214:5) name: { code: 'Custom_Warning', detail: 'Additional information about warning' } } (node:13011) [object Object]: An Error occured!
Example 2
Let’s take a look at one more example.
console.log("Start ..."); // Interval set to keep the process running setInterval(() => { console.log("Running ..."); }, 1000); setTimeout(() => { process.emitWarning('An Error occured!', { code: 'Custom_Warning', detail: 'Additional information about warning' }); }, 2000); setTimeout(() => { process.emitWarning('ALERT! WARNING OCCURED', { type: 'IMPORTANT', code: '001', detail: 'Can not proceed further!' }); }, 4000); // Start listening process.on('warning', (warning) => { // Print msg if there is an Important warning if (warning.name === 'IMPORTANT') { console.log('Fix this ASAP!') process.exit(0); } });
Output
Start ... Running ... Running ... Running ... Running ... Running ... Running ... Running ... Running ... Running ... (node:18756) [object Object]: An Error occured! (node:18756) [object Object]: ALERT! WARNING OCCURED
Advertisements