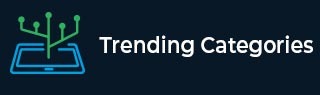
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Node.js - process.disconnect() Method
When a Node.js process is spawned with an IPC channel, the process.disconnect() method will close that IPC channel to the parent process, allowing the child process to exit or finish gracefully. The process will exit once there are no other connections keeping it alive.
Syntax
process.disconnect()
Example 1
Create two files with the names "parent.js" and "child.js" and copy the following code snippets. After creating the file, use the command "node parent.js" to run parent.js.
parent.js
// process.channel Property Demo Example // Importing the child_process modules const fork = require('child_process').fork; // Attaching the child process file const child_file = 'child.js'; // Spawning/calling child process const child = fork(child_file);
child.js
console.log('In Child Process') // Checking if channel is available if (process.connected) { // Check if its connected or not if (process.connected == true) { console.log("Child is connected."); } // Use process.disconnect() to disconnect with child process.disconnect(); // Check if its connected or not if (process.connected == false) { console.log("Child is now disconnected."); } }
Output
C:\home
ode>> node parent.js In Child Process Child is connected. Child is now disconnected.
Example 2
Let's take a look at one more example.
parent.js
// process.channel Property Demo Example // Importing the child_process modules const fork = require('child_process').fork; // Attaching the child process file const child_file = 'child.js'; // Spawning/calling child process const child = fork(child_file);
child.js
console.log('In Child Process') // Checking if channel is available if (process.connected) { // Send multiple messages setTimeout((function () { if (process.connected == true) { console.log("Process Running for: 1 second."); } }), 1000); // Disconnect from channel after 2 seconds setTimeout((function () { if (process.connected == true) { console.log("Process Running for: 2 seconds."); } }), 2000); // Disconnect from channel after 2.5 seconds setTimeout((function () { process.disconnect(); }), 2500); // Disconnect from channel after 3 seconds setTimeout((function () { if (process.connected == true) { console.log("Process Running for: 3 seconds."); } }), 3000); }
Output
C:\home
ode>> node parent.js In Child Process Process Running for: 1 second. Process Running for: 2 seconds.
Advertisements