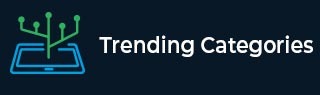
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Node.js – Monitor mode in Redis
Redis also supports the monitor command that lets the user to see all the commands received by the Redis server across all the client connections. These connections include commands from everywhere, including other client libraries and computers as well.
The monitor event will monitor all the commands which are executed on the Redis server where monitor is enabled. The callback from the monitor receives the timestamp from the Redis server, an array of commands along with the raw monitoring string.
Syntax
client.monitor( function(callback) )
Example 1
Create a file with the name "monitor.js" and copy the following code. After creating the file, use the command "node monitor.js" to run this code as shown in the example below:
// Monitor mode Demo Example // Importing the redis module const redis = require("redis"); // Creating redis client const client = redis.createClient(); // Stating that we are entering into the monitor mode client.monitor(function(err, res) { console.log("Entering monitoring mode."); }); // Setting value in redis client.set("hello", "tutorialspoint"); // Defining the monitor output client.on("monitor", function(time, args, rawReply) { console.log(time + ": " + args); // 1458910076.446514:['set', 'foo', 'bar'] });
Output
Entering monitoring mode. 1623087421.448855: set,hello,tutorialspoint
Example 2
Let's take another example −
// Monitor mode Demo Example // Importing the redis module const redis = require("redis"); // Creating redis client const client = redis.createClient(); // Stating that we are entering into the monitor mode client.monitor(function(err, res) { console.log("Entering monitoring mode."); }); // Setting value in redis client.set("hello", "tutorialspoint"); client.get("hello"); client.set("key", "value"); client.set("key2", "value2"); client.get("key2"); // Defining the monitor output client.on("monitor", function(time, args, rawReply) { console.log(time + ": " + args); // 1458910076.446514:['set', 'foo', 'bar'] });
Output
Entering monitoring mode. 1623088106.382888: set,hello,tutorialspoint 1623088106.382902: get,hello 1623088106.382919: set,key,value 1623088106.383023: set,key2,value2 1623088106.383033: get,key2
Advertisements