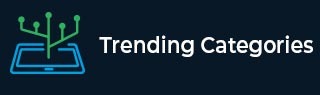
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Nim Game in C++
Suppose we are playing a game called, Nim Game with another player. There is a heap of stones, each time one player takes turns to remove 1 to 3 stones. The one who removes the last stone will be the winner. Player1 will take the first turn to remove the stones. Both of the players are very clever and have optimal strategies for the game. We have to devise an algorithm to determine whether player1 can win the game given the number of stones in the heap.
So, if the input is like 5, then the output will be true, as there are 5 stones, so at the beginning, if player1 takes one stone, then the second player can take 1 to 3 stones, there will be at least one stone left after player2's turn, so player1 can win.
This can be solved using one simple step −
return true when n mod 4 is not same as 0, otherwise false
Example
Let us see the following implementation to get a better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: bool canWinNim(int n) { return n%4!=0; } }; main(){ Solution ob; cout << (ob.canWinNim(5)); }
Input
5
Output
1