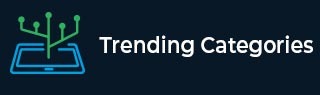
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
New Features of Java 12
Introduction to New Features of Java 12
On March 19, 2019, Java 12 was made available. Several new and enhanced features included in Java 12's release make it a worthwhile upgrade over Java 11 in almost every way. Switch Expressions, Default CDS Archives, Shenandoah, and Microbenchmark Suite are a few of the Java 12 features that deserve special mention.
To increase Java's productivity, usability, and versatility for coders, these features have been added. We'll talk in-depth about these new capabilities in this article.
Switch Expressions (JEP 325)
Something interesting to point out is that Java 12 has brought about a noteworthy enhancement in switch expressions. They can now be utilized as both statements and expressions, resulting in simplified code and allowing for pattern matching with the switch.
In the past, not including break statements could result in default fall through, a prevalent cause of errors in Java code. Nevertheless, with the latest update in Java 12, the default case is now compulsory in switch expressions, preventing such problems. These modifications in the switch expressions feature improve Java 12's efficiency and reliability as a programming language for developers.
Let us look at the traditional switch statement (Java 11) vs Java 12 through example code to have a better understanding.
Collectors.teeing() in Stream API
As a static approach, a teeing collector has been made available. Collectors::teeing. This collection sends its input to two additional collectors, who then combine their output with a function.
Two collectors can be provided to teeing(Collector, Collector, BiFunction), along with a function to combine the data. Both downstream collectors each process each element passed to the resulting collector, and the output of their processing is combined with the output of the final collector using the stated merge function.
Algorithm
Step 1 − Firstly, we need to create a stream of integer numbers between 8 to 14.
Step 2 − Next, we can use a collector built with the newly added Collectors.teeing() function in Java 12 to determine the average value of the integers in the stream.
Step 3 − The Collectors.teeing() method employs two collectors and a function that merges their outcomes to calculate the mean value.
Step 4 − Finally, the computed average is printed to the console for display.
Example 1
This program demonstrates how to use the Collectors.teeing() method to calculate the average value of a stream of integers.
import java.util.stream.Collectors; import java.util.stream.Stream; public class APITester { public static void main(String[] args) { double mean = Stream.of(8, 9, 10, 11, 12, 13, 14) .collect(Collectors.teeing( Collectors.summingDouble(i -> i), Collectors.counting(), (sum, n) -> sum / n)); System.out.println(mean); } }
Output
11.0
Shenandoah
Have you heard about Shenandoah? It's a new and enhanced Garbage Collector, although it's still in the experimental stage. This GC algorithm provides a low pause time by executing evacuation work concurrently with the running Java threads. This means that pause times are not dependent on the heap size. Whether you have a small 5MB heap or a massive 10GB one, the pause times will be the same.
JVM Constant API
If you're working with programs that manipulate classes and methods, the JVM constants API could be a valuable tool for you. In these types of programs, byte code instructions need to be modeled and loadable constants must be handled. While constants of the String or Integer type usually present no issues, those of the Class type can be more challenging. Loading classes can fail if they're inaccessible or non-existent.
The good news is that the new API simplifies matters by using interfaces like ClassDesc, MethodTypeDesc, MethodHandleDesc, and DynamicConstantDesc to symbolically handle constant values. This approach eliminates much of the complexity involved in working with class constants, making it easier to manipulate and manage them in your programs.
Default CDS Archives
Class Data Sharing (CDS) is an essential feature that reduces the startup time and memory footprint across multiple Java Virtual Machines. It achieves this by utilizing a default class list generated at build-time, which contains the essential core library classes.
In Java 12, there has been a change to this feature - the CDS archive is now enabled by default. To disable CDS and run programs without it, we need to manually set the Xshare flag to "off".
Abortable Mixed Collections for G1(JEP 344)
The Garbage First (G1) garbage collector, which is set as the default, boasts an impressive analysis engine that determines the collection set. Once the process is initiated, it's designed to collect all live objects without interruption. However, this method can occasionally lead to performance issues when the target pause time is exceeded.
To address this problem, G1 collection sets are now separated into optional and mandatory sections, allowing for the process to be abortable. Prioritizing the mandatory set can often ensure that the target pause time is achieved, preventing the collection process from exceeding its limit. This technique is critical in enabling the G1 garbage collector to operate efficiently, without causing any significant performance issues.
Microbenchmark Suite(JEP 230)
This latest addition is a game-changer for developers who can now seamlessly create and execute microbenchmarks with ease. Microbenchmarks are a pivotal tool used to measure the effectiveness of individual code snippets or methods. They help pinpoint performance hurdles and enhance code to maximize efficiency.
With the newly launched Microbenchmark Suite, developers can now effortlessly run pre-existing microbenchmarks or curate new ones with minimal exertion. The suite is intelligently integrated into the JDK source code, which means that developers can benchmark their code seamlessly.
Default Class Data Sharing Archives(JEP 341)
A CDS (Default Class Data Sharing) archive creation procedure has been enhanced in Java 12. The JDK build procedure on the 64-bit platform uses the default class list to accomplish this. As a result, the procedure for creating CDS archives is improved and streamlined.
Promptly Return Unused Committed Memory from G1(JEP 346)
The G1 garbage collector in Java 12 can now quickly return underutilized committed memory to the operating system thanks to JEP 346. The G1 garbage collection couldn't return Java heap memory to the OS when idle in Java 11, so this is an upgrade. Because of the G1 garbage collector, Java 12 provides enhanced memory management abilities, resulting in the more effective use of system resources.
Files.mismatch() Method
Introducing "Files.mismatch()," a novel technique that enables file comparisons. The signature of this useful function is "public static long mismatch(Path path1, Path path2) throws IOException," and it gives -1L if there are no mismatches. The first one's location will be provided if there is a mismatch, though.
Two distinct scenarios could result in mismatches −
First, if the file sizes vary, the method will return the smaller file size.
Furthermore, it will report the position of the first different byte if the files' bytes don't match.
Conclusion
Java 12 boasts new features that enhance programming for developers. With Switch expressions, collectors.teeing() in Stream API, and Shenandoah, Java is now more efficient and reliable. The JVM Constant API, Default CDS archives, Abortable Mixed Collections for G1, and Microbenchmark suite make Java more productive and versatile. These upgrades simplify code and increase application robustness.