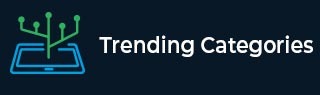
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Name the different locators used in Selenium with python.
The different locators used in Selenium with python are listed below.
Id - The element is identified with its id attribute. NoSuchElementException raised if element with matching id is not available.
Syntax −
driver.find_element_by_id("id")
Name - The element is identified with its name attribute. NoSuchElementException raised if element with matching name is not available.
Syntax −
driver.find_element_by_name("name")
Xpath - The element is identified with the attributes and tagname. There are two types of xpath – absolute and relative.
Syntax −
driver.find_element_by_xpath("//input[@type='type']")
CSS - The element is identified with the help of css expression built with the help of attributes like id, class and tagName. NoSuchElementException raised if element with matching css is not available.
Syntax −
driver.find_element_by_css_selector("#id")
Link Text - The element is identified with its link text enclosed in anchor tag. NoSuchElementException raised if element with matching link text is not available.
Syntax −
driver.find_element_by_link_text("Home Link")
Partial Link Text - The element is identified by matching partially with its link text enclosed in anchor tag. NoSuchElementException raised if element with matching partial link text is not available.
Syntax −
driver.find_element_by_partial_link_text("Home")
Tagname – The element is identified by its tagname. NoSuchElementException is raised if the element has no matching tagname.
Syntax −
driver.find_element_by_tag_name("a")
Class name - The element is identified with its name of the class attribute. NoSuchElementException raised if element with matching class name attribute is not available.
Syntax −
driver.find_element_by_class_name("search")
Example
Code Implementation with id locator.
from selenium import webdriver #browser exposes an executable file #Through Selenium test we will invoke the executable file which will then #invoke actual browser driver = webdriver.Chrome(executable_path="C:\chromedriver.exe") # to maximize the browser window driver.maximize_window() #get method to launch the URL driver.get("https://www.tutorialspoint.com/index.htm") #to refresh the browser driver.refresh() # identifying the edit box with the help of id driver.find_element_by_id("gsc-i-id1").send_keys("Selenium") #to close the browser driver.close()