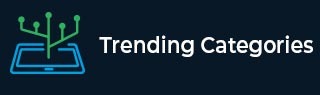
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
N consecutive odd numbers JavaScript
In this problem statement, our target is to print the n consecutive odd numbers and implement this problem with the help of Javascript functionalities. So we can solve this problem with the help of loops in Javascript.
Logic for the given problem
In the given problem statement we have to design a program to generate a given number of consecutive odd numbers starting from 1. It will be done by creating an empty array to store the odd numbers and then using a loop which will run n times to add every odd number to the created array.
The loop will start with the first odd number which is 1 and increment by 2 to get the forthcoming odd number. If the loop will finish, the function will return the array which is containing the n consecutive odd numbers.
Algorithm
Step 1 − In this program, we need to generate n consecutive odd numbers so first we need to assign it to a variable called n.
Step 2 − As we know, the first odd number is always 1 so we will assign it to another variable called begin.
Step 3 − Now we will create a blank array called oddNums which is used to store the sequence of consecutive odd numbers.
Step 4 − After defining the above things, we need to loop through the sequence of numbers n times. For every traverse of the loop we will add the current value of begin to the oddNums array. And then increment the value of begin by 2 to get the next odd number in the sequence.
Step 5 − When the loop gets finished we will return the oddNums array which is now containing the sequence of odd numbers till n.
Code for the algorithm
//function for getting n consecutive odd numbers function consecutiveOddNumbers(n) { let begin = 1; const oddNums = []; for (let i = 0; i < n; i++) { oddNums.push(begin); begin += 2; } return oddNums; } console.log(consecutiveOddNumbers(7));
Complexity
The time complexity for the algorithm is O(n). Because the loop is running n times and every iteration is taking a constant amount of time to append an item to the array and increment the begin variable by 2. The space complexity for the code is O(n) because we have created an array of size n to store the odd numbers.
Conclusion
As we have seen the code for implementing the sequence of odd numbers for n numbers starting from 1 and returns them as an array. We have used basic Javascript functionality to produce the code. The time complexity for the algorithm is linear which is an efficient solution for creating odd numbers. But for very large n values the space complexity will become less efficient.