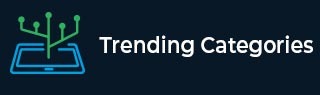
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
multiset count() function in C++ STL
In this article we will be discussing the working, syntax and examples of multiset::count() function in C++ STL.
What is a multiset in C++ STL?
Multisets are the containers similar to the set container, meaning they store the values in the form of keys same like a set, in a specific order.
In multiset the values are identified as keys as same as sets. The main difference between multiset and set is that the set has distinct keys, meaning no two keys are the same, in multiset there can be the same keys value.
Multiset keys are used to implement binary search trees.
What is multiset::count()?
multiset::count() function is an inbuilt function in C++ STL, which is defined in <set> header file.
This function counts the number of the elements with a specific key.
A multiset can have multiple values of the same key so when we want to count the number of the values of the same key we can use count(). count() searches for the key in the whole container and returns the results. If there are no keys we are looking for in the container then the function returns 0.
Syntax
ms_name.count(value_type T);
Parameters
The function accepts one parameter of the multiset’s value type, which we have to search for in the multiset container associated.
Return value
This function returns the number values present with the same key.
Example
Input: std::multiset<int> mymultiset = {1, 2, 2, 3, 2, 4}; mymultiset.count(2); Output: 3
Example
#include <bits/stdc++.h> using namespace std; int main() { int arr[] = {1, 2, 3, 1, 1, 1}; multiset<int> check(arr, arr + 6); cout<<"List is : "; for (auto i = check.begin(); i != check.end(); i++) cout << *i << " "; cout << "\n1 is occuring: "<<check.count(1)<<" times"; return 0; }
Output
If we run the above code it will generate the following output −
List is : 1 1 1 1 2 3 1 is occuring 4 times
Example
#include <bits/stdc++.h> using namespace std; int main() { int arr[] = {1, 2, 3, 1, 1, 1, 2, 2}; multiset<int> check(arr, arr + 8); cout<<"List is : "; for (auto i = check.begin(); i != check.end(); i++) cout << *i << " "; cout << "\n1 is occuring: "<<check.count(1)<<" times"; cout << "\n2 is occuring: "<<check.count(2)<<" times"; return 0; }
Output
If we run the above code it will generate the following output −
List is : 1 1 1 1 2 2 2 3 1 is occuring 4 times 2 is occuring 3 times