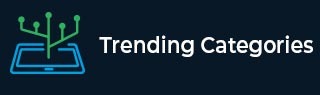
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum swaps required between two strings to make one string strictly greater than the other
In this article, we'll discuss an intriguing problem in string manipulation - "Minimum swaps required between two strings to make one string strictly greater than the other". We'll understand the problem, detail a strategy to solve it, implement it in C++, and clarify the concept with a relevant example.
Understanding the Problem Statement
Given two strings of equal length, our goal is to determine the minimum number of character swaps required to make one string strictly greater than the other. The characters are swapped between the two strings, and each swap operation involves exactly one character from each string. The strings are compared lexicographically, where 'a' < 'b' < 'c', and so forth.
Approach
The idea is to use a greedy algorithm. We start from the beginning of the strings and, for each position, if the character in the first string is smaller than the corresponding character in the second string, we swap them. If they're equal, we look for a larger character in the second string to swap with. If no such character is found, we continue to the next position. We repeat this until we've processed all characters in the strings.
Example
Let's implement the above approach −
#include <stdio.h> #include <string.h> int minSwaps(char* s1, char* s2) { int swaps = 0; int n = strlen(s1); for (int i = 0; i < n; i++) { if (s1[i] < s2[i]) { char temp = s1[i]; s1[i] = s2[i]; s2[i] = temp; swaps++; } else if (s1[i] == s2[i]) { for (int j = i + 1; j < n; j++) { if (s2[j] > s1[i]) { char temp = s1[i]; s1[i] = s2[j]; s2[j] = temp; swaps++; break; } } } } return (strcmp(s1, s2) > 0) ? swaps : -1; } int main() { char s1[] = "bbca"; char s2[] = "abbc"; int swaps = minSwaps(s1, s2); if (swaps != -1) printf("Minimum swaps: %d\n", swaps); else printf("Cannot make string 1 greater\n"); return 0; }
Output
Minimum swaps: 2
#include<bits/stdc++.h> using namespace std; int minSwaps(string &s1, string &s2) { int swaps = 0; int n = s1.size(); for(int i=0; i<n; i++) { if(s1[i] < s2[i]) { swap(s1[i], s2[i]); swaps++; } else if(s1[i] == s2[i]) { for(int j=i+1; j<n; j++) { if(s2[j] > s1[i]) { swap(s1[i], s2[j]); swaps++; break; } } } } return (s1 > s2) ? swaps : -1; } int main() { string s1 = "bbca"; string s2 = "abbc"; int swaps = minSwaps(s1, s2); if(swaps != -1) cout << "Minimum swaps: " << swaps << "\n"; else cout << "Cannot make string 1 greater\n"; return 0; }
Output
Minimum swaps: 2
public class Main { public static int minSwaps(String s1, String s2) { int swaps = 0; int n = s1.length(); char[] s1Arr = s1.toCharArray(); char[] s2Arr = s2.toCharArray(); for (int i = 0; i < n; i++) { if (s1Arr[i] < s2Arr[i]) { char temp = s1Arr[i]; s1Arr[i] = s2Arr[i]; s2Arr[i] = temp; swaps++; } else if (s1Arr[i] == s2Arr[i]) { for (int j = i + 1; j < n; j++) { if (s2Arr[j] > s1Arr[i]) { char temp = s1Arr[i]; s1Arr[i] = s2Arr[j]; s2Arr[j] = temp; swaps++; break; } } } } return (new String(s1Arr).compareTo(new String(s2Arr)) > 0) ? swaps : -1; } public static void main(String[] args) { String s1 = "bbca"; String s2 = "abbc"; int swaps = minSwaps(s1, s2); if (swaps != -1) System.out.println("Minimum swaps: " + swaps); else System.out.println("Cannot make string 1 greater"); } }
Output
Minimum swaps: 2
def min_swaps(s1, s2): swaps = 0 n = len(s1) s1 = list(s1) s2 = list(s2) for i in range(n): if s1[i] < s2[i]: s1[i], s2[i] = s2[i], s1[i] swaps += 1 elif s1[i] == s2[i]: for j in range(i + 1, n): if s2[j] > s1[i]: s1[i], s2[j] = s2[j], s1[i] swaps += 1 break return swaps if ''.join(s1) > ''.join(s2) else -1 s1 = "bbca" s2 = "abbc" swaps = min_swaps(s1, s2) if swaps != -1: print("Minimum swaps:", swaps) else: print("Cannot make string 1 greater")
Output
Minimum swaps: 2
Test Case
Let's consider the strings "bbca" and "abbc". The following swaps would occur −
Swap 'b' in the first string with 'a' in the second string. The strings now are "bbac" and "abbc".
Swap 'c' in the first string with 'b' in the second string. The strings now are "bbcb" and "abac".
"bbcb" is lexicographically greater than "abac". Therefore, the minimum number of swaps required is 2, and the output of the program will be "Minimum swaps: 2".
Conclusion
In this article, we explored the problem of determining the minimum number of swaps required between two strings to make one string lexicographically greater than the other. We discussed a strategy to solve the problem and explained the concept with an example. String manipulation problems like these are common in interviews and competitive programming, and understanding these concepts can be quite beneficial.