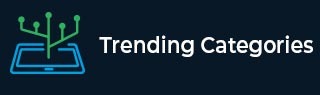
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum String in C++
Suppose we have two strings s and t of same length, and both are in lowercase letters. Consider we have rearranged s at first into any order, then count the minimum number of changes needed to turn s into t.
So, if the input is like s = "eccynue", t = "science", then the output will be 2 as if we rearrange "eccynue" to "yccence", then replace y with s and second c with i, it will be "science".
To solve this, we will follow these steps −
ret := 0
Define two arrays cnt1 to hold frequency of s and cnt2 to hold frequency of t
for initialize i := 0, when i < 26, update (increase i by 1), do −
ret := ret + max(cnt1[i] - cnt2[i], 0)
return ret
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: int solve(string s, string t) { int ret = 0; vector <int> cnt1(26); vector <int> cnt2(26); for(int i = 0; i < s.size(); i++){ cnt1[s[i] - 'a']++; } for(int i = 0; i < t.size(); i++){ cnt2[t[i] - 'a']++; } for(int i = 0; i < 26; i++){ ret += max(cnt1[i] - cnt2[i], 0); } return ret; } }; int main(){ Solution ob; cout << (ob.solve("eccynue", "science")); }
Input
"eccynue", "science"
Output
2
Advertisements