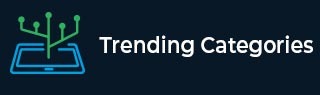
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum Size of Two Non-Overlapping Intervals in C++
Suppose we have a list of intervals where each interval contains the [start, end] times. We have to find the minimum total size of any two non-overlapping intervals, where the size of an interval is (end - start + 1). If we cannot find such two intervals, return 0.
So, if the input is like [[2,5],[9,10],[4,6]], then the output will be 5 as we can pick interval [4,6] of size 3 and [9,10] of size 2.
To solve this, we will follow these steps −
ret := inf
n := size of v
sort the array v based on the end time
Define an array dp of size n
for initialize i := 0, when i < size of v, update (increase i by 1), do −
low := 0, high := i - 1
temp := inf
val := v[i, 1] - v[i, 0] + 1
while low <= high, do
mid := low + (high - low) / 2
if v[mid, 1] >= v[i, 0], then −
high := mid - 1
Otherwise
temp := minimum of temp and dp[mid]
low := mid + 1
if temp is not equal to inf, then −
ret := minimum of ret and (temp + val)
dp[i] := minimum of val and temp
Otherwise
dp[i] := val
if i > 0, then
dp[i] := minimum of dp[i] and dp[i - 1]
return (if ret is same as inf, then 0, otherwise ret)
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: static bool cmp(vector <int>& a, vector <int>& b){ return a[1] < b[1]; } int solve(vector<vector<int>>& v) { int ret = INT_MAX; int n = v.size(); sort(v.begin(), v.end(), cmp); vector <int> dp(n); for(int i = 0; i < v.size(); i++){ int low = 0; int high = i - 1; int temp = INT_MAX; int val = v[i][1] - v[i][0] + 1; while(low <= high){ int mid = low + (high - low) / 2; if(v[mid][1] >= v[i][0]){ high = mid - 1; }else{ temp = min(temp, dp[mid]); low = mid + 1; } } if(temp != INT_MAX){ ret = min(ret, temp + val); dp[i] = min(val, temp); }else{ dp[i] = val; } if(i > 0) dp[i] = min(dp[i], dp[i - 1]); } return ret == INT_MAX ? 0 : ret; } }; main(){ Solution ob; vector<vector<int>> v = {{2,5},{9,10},{4,6}}; cout << (ob.solve(v)); }
Input
{{2,5},{9,10},{4,6}}
Output
5