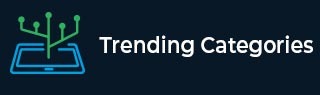
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum number of swaps required to sort an array in C++
Problem statement
Given an array of N distinct elements, find the minimum number of swaps required to sort the array
Example
If array is {4, 2, 1, 3} then 2 swaps are required
- Swap arr[0] with arr[2]
- Swap arr[2] with arr[3}
Algorithm
1. Create a vector of pair in C++ with first element as array alues and second element as array indices. 2. Sort the vector of pair according to the first element of the pairs. 3. Traverse the vector and check if the index mapped with the value is correct or not, if not then keep swapping until the element is placed correctly and keep counting the number of swaps.
Example
#include <bits/stdc++.h> using namespace std; int getMinSwaps(int *arr, int n) { vector<pair<int, int>> vec(n); for (int i = 0; i < n; ++i) { vec[i].first = arr[i]; vec[i].second = i; } sort(vec.begin(), vec.end()); int cnt = 0; for (int i = 0; i < n; ++i) { if (vec[i].second == i) { continue; } swap(vec[i].first,vec[vec[i].second].first); swap(vec[i].second,vec[vec[i].second].second); if (i != vec[i].second) { --i; } ++cnt; } return cnt; } int main() { int arr[] = {4, 2, 1, 3}; int n = sizeof(arr) / sizeof(arr[0]); cout << "Minimum swaps = " << getMinSwaps(arr, n) << endl; return 0; }
When you compile and execute above program. It generates following output
Output
Minimum swaps = 2
Advertisements