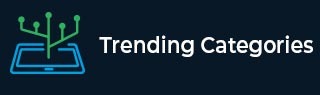
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum number of elements that should be removed to make the array good using C++.
Problem statement
Given an array “arr”, the task is to find the minimum number of elements to be removed to make the array good.
A sequence a1, a2, a3. . .an is called good if for each element a[i], there exists an element a[j] (i not equals to j) such that a[i] + a[j] is a power of two.
arr1[] = {1, 1, 7, 1, 5}
In above array if we delete element ‘5’ then array becomes good array. After this any pair of arr[i] + arr[j] is power of two −
- arr[0] + arr[1] = (1 + 1) = 2 which power of two
- arr[0] + arr[2] = (1 + 7) = 8 which is power of two
Algorithm
1. We have to delete only such a[i] for which there is no a[j] such that a[i] + a[i] is a power of 2. 2. For each value find the number of its occurrences in the array 3. Check that a[i] doesn’t have a pair a[j]
Example
#include <iostream> #include <map> #define SIZE(arr) (sizeof(arr) / sizeof(arr[0])) using namespace std; int minDeleteRequred(int *arr, int n){ map<int, int> frequency; for (int i = 0; i < n; ++i) { frequency[arr[i]]++; } int delCnt = 0; for (int i = 0; i < n; ++i) { bool doNotRemove = false; for (int j = 0; j < 31; ++j) { int pair = (1 << j) - arr[i]; if (frequency.count(pair) && (frequency[pair] > 1 || (frequency[pair] == 1 && pair != arr[i]))) { doNotRemove = true; break; } } if (!doNotRemove) { ++delCnt; } } return delCnt; } int main(){ int arr[] = {1, 1, 7, 1, 5}; cout << "Minimum elements to be deleted = " << minDeleteRequred(arr, SIZE(arr)) << endl; return 0; }
Output
When you compile and execute above program. It generates following output −
Minimum elements to be deleted = 1
Advertisements