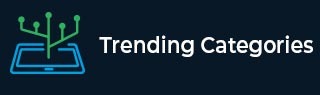
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum Number of Adjacent Awaps to Convert a String into its given Anagram
Every string is formed by a sequence of characters arranged in an order. The string may be composed of letters, number or even special characters.
An anagram of any input string, is the string with a random permutation of characters. This implies, that when the order of the characters is rearranged, an anagram of the string is obtained. The respective counts of the characters should also remain the same in anagrams. Two anagram strings have the following implications −
Both of them contains the same set of characters.
Both of them may have a different permutation of characters
For instance, feed and def are not anagrams of each other, since the character ‘e’ in the first string is repeated twice.
Adjacent characters are the neighbouring positioned letters in a string.
In order to equalise the strings, the corresponding characters of both the strings have to be matched.
The problem statement is to first check whether the given two strings are anagram of each other and return the minimum of swaps required to obtain equal anagram strings, if they are.
Some of the examples illustrating the problem statement are as follows −
Sample Example
Example 1 - str1 : “abca”
str2 : “baac”
Output : 2
Explanation : The following strings are anagrams of each other, and the str1 can be converted to str2 by performing the following two swaps −
swap(0,1) which yields “baca”
swap(2,3) which yields “baac”
Example 2 - str1 : “aaf”
str2 : “faa”
Explanation : 2
Initially, the swap(2,3) is performed to yield “afa”
Then, swap(1,2) is performed to yield “faa”
This problem can be solved by using the concepts of character checking and using STL inbuilt-methods −
sort() to sort the string
swap(i,j) to swap the characters at the i and jth index positions respectively.
Algorithm
Step 1 − The provided two strings, str1 and str2 are sorted using the sort() method.
Step 2 − Their characters are respectively compared to check if the two strings are equivalent in nature.
Step 3 −If the two sorted strings are equivalent then they are anagram of each other.
Step 4 −A counter is maintained to keep a track on the number of swaps performed until now.
Step 5 − Two pointers, i and j are initialised and the pointer owing to the second string is incremented until the character at the ith index of the first string and jth index of second string match, such that str1[i] = str2[j].
Step 6 − In order to match the positions of equal characters, the adjacent elements and the indices j and j-1 are interchanged using the swap() function.
Step 7 − The j counter is then decremented till it becomes equivalent to i.
Step 8 − The i pointer is now incremented.
Step 9 − This procedure follows until the entire length is exhausted.
Example
The following C++ code snippet takes as input two strings, and finds out if these two strings are anagrams of each other and the least number of swaps to convert them to equivalent strings
//including the required libraries #include <bits/stdc++.h> using namespace std; //check whether the two input strings are anagram of each other or not bool checkanagram(string s1, string s2) { //sorting the strings to check the equivalence of strings after sorting sort(s1.begin(), s1.end()); sort(s2.begin(), s2.end()); //checking if all the characters occur in order if (s1 == s2) return true; else return false; } // Function to return the minimum swaps required int minSwaps(string str1, string str2) { //initialising the pointers int i = 0, j = 0; //initialising minswaps with 0 int minswaps = 0; int len = str1.length(); //changing the characters of str1 to be equivalent to str2 for(i=0; i < len;i++) { //initialising the second pointer at the first one j = i; //computing the index element where jth index of first string is equivalent to ith index of second string while (str1[j] != str2[i]) { j += 1; } //equalising element available at the ith position while (i < j) { //swapping adjacent elements available at the j and j-1 positions respectively swap(str1[j],str1[j-1]); //increasing the number of swaps each time minswaps += 1; j--; } } return minswaps; } //calling the method to simulate minimum swaps for conversion of strings int main() { //declaring both the strings string str1 = "male"; string str2 = "lame"; //check if both the strings are anagram of each other bool anares = checkanagram(str1,str2); if(anares){ int swaps = minSwaps(str1,str2); cout<<"Minimum no of swaps to convert string1 to string2 : "<<swaps; } else cout << "Strings are not anagram of each other."; return 0; }
Output
Minimum no of swaps to convert string1 to string2 : 3
Conclusion
The anagram strings are just different permutations of the same set of characters. They can be made similar by just swapping the characters at the corresponding positions.
The time complexity of the above approach is O(n*n), where n is the length of the string. This is because each index character in the first string is searched for in the entire length of second string.
The space complexity of the above specified algorithm is O(1), which is constant in nature.