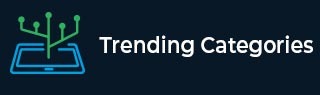
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum height of a triangle with given base and area in C++
Description
Given two integers a and b, find the smallest possible height such that a triangle of atleast area ‘a’ and base ‘b’ can be formed.
Example
If a = 16 and b = 4 then minimum height would be 8
Algorithm
Area of triangle can be calculate using below formula −
area = ½ * height * base
Using above formula, height can be calculated as −
height = (2 * area) / base
So Minimum height is the ceil() of the height obtained using above formula.
Example
#include <iostream> #include <cmath> using namespace std; float minHeight(int area, int base) { return ceil((2 * area) / base); } int main() { int area = 16, base = 4; cout << "Minimum height = " << minHeight(area, base) << endl; return 0; }
Output
When you compile and execute above program. It generates following output −
Minimum height = 8
Advertisements