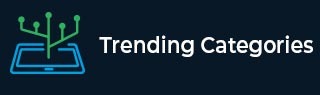
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Minimum Flips to Make a OR b Equal to c in C++
Suppose we have 3 positives numbers a, b and c. We have to find the minimum flips required in some bits of a and b to make (a OR b == c ). Here we are considering bitwise OR operation.
The flip operation consists of change any single bit 1 to 0 or change the bit 0 to 1 in their binary representation. So if a : 0010 and b := 0110, so c is 0101, After flips, a will be 0001, and b will be 0100
To solve this, we will follow these steps −
- ans := 0
- for i in range 0 to 31
- bitC := (c / 2^i) AND 1
- bitA := (a / 2^i) AND 1
- bitB := (b / 2^i) AND 1
- if (bitA OR bitB) is not same as bitC, then
- if bitC is 0
- if bitA = 1 and bitB = 1, then increase ans by 2, otherwise increase ans by 1
- otherwise increase ans by 1
- if bitC is 0
- return ans
Example(C++)
Let us see the following implementation to get a better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: int minFlips(int a, int b, int c) { int ans = 0; for(int i = 0; i < 32; i++){ int bitC = (c >> i) & 1; int bitA = (a >> i) & 1; int bitB = (b >> i) & 1; if((bitA || bitB) != bitC){ if(!bitC){ if(bitA == 1 && bitB == 1){ ans += 2; } else { ans += 1; } } else{ ans += 1; } } } return ans; } }; main(){ Solution ob; cout << (ob.minFlips(2,6,5)); }
Input
2 6 5
Output
3
Advertisements