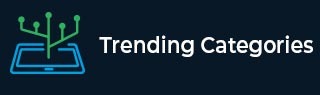
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Method Overloading and null error in Java
When method is overloaded in Java, the functions have the same name, and the number of parameters to the function is same. In such cases, if the parameters are non-primitive and have the ability to accept null values, the compiler gets confused when the function is called with a null value, since it can’t choose either of them, because both of them have the ability to accept null values. This results in a compile time error.
Example
Below is an example showing the same −
public class Demo { public void my_function(Integer i) { System.out.println("The function with integer as parameter is called "); } public void my_function(String name) { System.out.println("The function with string as parameter is called "); } public static void main(String [] args) { Demo my_instance = new Demo(); my_instance.my_function(null); } }
Output
/Demo.java:15: error: reference to my_function is ambiguous my_instance.my_function(null); ^ both method my_function(Integer) in Demo and method my_function(String) in Demo match 1 error
The solution in such cases has been demonstrated below −
Example
public class Demo { public void my_function(Integer i) { System.out.println("The function with integer as parameter is called "); } public void my_function(String name) { System.out.println("The function with string as parameter is called "); } public static void main(String [] args) { Demo my_instance = new Demo(); String arg = null; my_instance.my_function(arg); } }
Output
The function with string as parameter is called
A class named Demo contains a function named ‘my_function’ which takes an integer as parameter. The same function is overloaded, and the parameter is a string. Relevant messages are printed on the screen when any of these two functions is called. In the main function, an instance of the Demo class is created, and an argument of string type is assigned a null value. Now, this instance is called and the argument previously defined is passed as a parameter.