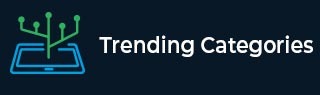
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Merge objects in array with similar key JavaScript
Let’s say, we have the following array of objects −
const arr = [ {id: 1, h1: 'Daily tests'}, {id: 2, h1: 'Details'}, {id: 1, h2: 'Daily classes'}, {id: 3, h2: 'Results'}, {id: 2, h3: 'Admissions'}, {id: 1, h4: 'Students'}, {id: 2, h5: 'Alumni'}, {id: 3, h3: 'Appreciations'}, {id: 1, h5: 'Tiny Tots'}, {id: 1, h6: 'Extras'}, ];
We have to write a function that converts this array into an array where all the headings (h1, h2, h3 …) that have the same id get clubbed inside the same object. Therefore, let’s write the code for this function −
Example
const arr = [ {id: 1, h1: 'Daily tests'}, {id: 2, h1: 'Details'}, {id: 1, h2: 'Daily classes'}, {id: 3, h2: 'Results'}, {id: 2, h3: 'Admissions'}, {id: 1, h4: 'Students'}, {id: 2, h5: 'Alumni'}, {id: 3, h3: 'Appreciations'}, {id: 1, h5: 'Tiny Tots'}, {id: 1, h6: 'Extras'}, ]; const clubArray = (arr) => { return arr.reduce((acc, val, ind) => { const index = acc.findIndex(el => el.id === val.id); if(index !== -1){ const key = Object.keys(val)[1]; acc[index][key] = val[key]; } else { acc.push(val); }; return acc; }, []); }; console.log(clubArray(arr));
Output
The output in the console will be −
[ { id: 1, h1: 'Daily tests', h2: 'Daily classes', h4: 'Students', h5: 'Tiny Tots', h6: 'Extras' }, { id: 2, h1: 'Details', h3: 'Admissions', h5: 'Alumni' }, { id: 3, h2: 'Results', h3: 'Appreciations' } ]
Advertisements