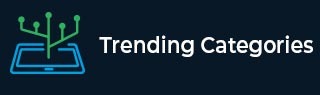
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Median after K additional integers in C++
In this problem, we are given an array of n integers and we are adding K elements to the array and then find the median of the resultant array. Given the condition, N+k is odd.
Let’s take an example to understand the problem,
Input −
array = {23, 65, 76, 67} ; k =1
Output −
67
To solve this problem, we will sort the given elements in ascending order and then add k elements at the end of the array i.e. we will take k greater elements.
The condition is given that n+k is odd. So, the median can be calculated using the formula, (n+k)/2.
Example
Program to find the median,
#include <bits/stdc++.h> using namespace std; int findMedianAfterK(int arr[], int n, int K) { sort(arr, arr + n); return arr[((n + K)/2)]; } int main() { int array[] = {3,56, 8, 12, 67, 10 }; int k = 3; int n = sizeof(array) / sizeof(array[0]); cout<<"The median after adding "<<k<<" elements is "<<findMedianAfterK(array, n, k); return 0; }
Output
The median after adding 3 elements is 56
Advertisements