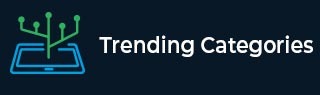
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum Width Ramp in C++
Suppose we have an array A of integers, a ramp is a tuple (i, j) for which i < j and A[i] <= A[j]. The width of such a ramp is j - i. We have to find the maximum width of a ramp in A. If one doesn't exist, then return 0. So if the input is like [6,0,8,2,1,5], then the output will be 4. Here the maximum width ramp is achieved at (i, j) = (1, 5) and A[1] = 0 and A[5] = 5
To solve this, we will follow these steps −
Create an array v, set n := size of the given array, set ret := 0
define a stack st
for i in range 0 to n – 1
if st is empty or the stack top element > A[i], then insert i into st
for i := n – 1 down to ret + 1
while st is not empty and top of st <= A[i]
ret := max of ret and (i – top of st)
delete from st
delete from st
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: int maxWidthRamp(vector<int>& A) { vector < pair <int, int> > v; int n = A.size(); int ret = 0; stack <int> st; for(int i = 0; i < n; i++){ if(st.empty() || A[st.top()] > A[i]){ st.push(i); } } for(int i = n - 1; i > ret; i--){ while(!st.empty() && A[st.top()] <= A[i]){ ret = max(ret, i - st.top()); st.pop(); } } return ret; } }; main(){ vector<int> v1 = {6,0,8,2,1,5}; Solution ob; cout << (ob.maxWidthRamp(v1)); }
Input
[6,0,8,2,1,5]
Output
4