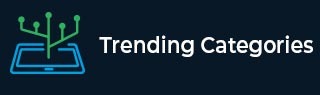
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum value of XOR among all triplets of an array in C++
In this problem, we are given an array of integers. Our task is to create the maximum value of XOR among all triplets of an array.
Let’s take an example to understand the problem,
Input − array = {5, 6, 1, 2}
Output − 6
Explanation −
All triplets are: 5^6^1 = 2 5^6^2 = 1 5^1^2 = 6 6^1^2 = 5
To solve this problem, a direct approach will be to find the XOR of all possible triplets and print the maximum of all triplets. This will not be effective if we work with an array with a huge number of elements in the array.
To find the maximum XOR, we will first create a set that contains the XOR of all pairs of elements. Then we will find the XOR between all pairs and the element and find the maximum XOR’s.
Example
Program to illustrate the working of our solution,
#include <bits/stdc++.h> using namespace std; int MaxTripletXor(int n, int a[]){ set<int> XORpair; for (int i = 0; i < n; i++) { for (int j = i; j < n; j++) { XORpair.insert(a[i] ^ a[j]); } } int maxXOR = 0; for (auto i : XORpair) { for (int j = 0; j < n; j++) { maxXOR = max(maxXOR, i ^ a[j]); } } return maxXOR; } int main(){ int matrix[] = {1, 2, 3, 5, 7}; int n = sizeof(matrix) / sizeof(matrix[0]); cout<<"The maximum XOR sum triplets is "<<MaxTripletXor(n, matrix); return 0; }
Output
The maximum XOR sum triplets is 7
Advertisements