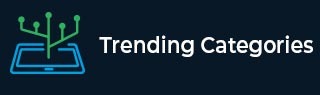
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum value of an integer for which factorial can be calculated on a machine in C++
In this problem, we need to create a program to find Maximum value of an integer for which factorial can be calculated on a machine in C++.
Factorial of a number is a huge value, as it is the product of all values preceding it. And C++ can handle large values only upto a certain value by using its inbuilt function. We need to find this restriction.
Solution Approach
We will simply use the property of data types which is when the numbers exceed the maximum value a negative number is returned.
We will use long long int which is the largest basic data type.
Example
#include <iostream> using namespace std; int calcMaxFactVal(){ int maxVal = 1; long long int maxFactorial = 1; while (true){ if (maxFactorial < 0) return (maxVal - 1); maxVal++; maxFactorial *= maxVal; } return - 1; } int main(){ cout<<"The maximum value of an integer for which factorial can be calculated on machine is "<<calcMaxFactVal(); return 0; }
Output
The maximum value of an integer for which factorial can be calculated on machine is 20
Advertisements