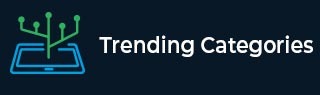
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum sum of distinct numbers with LCM as N in C++
In this article, we are given a number N. Our task is to create a program to find the maximum sum of distinct numbers with LCM as N in C++. To achive this first of all we need to find the sum of maximum numbers that have N as the Lowest Common Multiple (LCM).
For better understanding Let us go through 3 key concepts −
- Least Common Multiple (LCM): The lowest common multiple(LCM) of two or more integers is the smallest positive integer that is divisible for all of the integers. For example, the LCM of 4 and 5 is 20. Indeed, 20 is the smallest number that both 4 and 5 are divisible into without any remainders.
- Distinct Numbers: The numbers required to form the sum should not have any repetition (unique number). It means you cannot select a repeating number.
- Maximum Sum: You have to find the collection of unique numbers with the condition that their sum value is as big as possible and their LCM is still equal to N.
Following is the input and output scenario to find the maximum sum of dintinct numbers with LCM as N in C++.
Input
N = 18
Output
39
Explanation
LCM of the value N = 18 is 21 * 32.
The unique divisors (distinct numbers) of the value N = 18 are 1, 2, 3, 6, 9, 18.
Maximum sum with LCM 18 is 1 + 2 + 3 + 6 + 9 + 18 = 39.
Solution Approach
A simple solution to the problem would be using the idea that if we want the number N as LCM, then we need to take all the distinct divisors of N. And add them up to get the maxSum.
For this we will find all the factors of N. And then add them up, this will be given the maximum as we have considered all the numbers that can contribute to the LCM being N.
Example
Following is the program to find the maximum sum of distinct numbers with LCM as N in C++.
#include <iostream> using namespace std; int calcFactorSum(int N){ int maxSum = 0; for (int i = 1; i*i <= N; i++){ if (N % i == 0) { if (i == (N/i)) maxSum = maxSum + i; else maxSum = maxSum + i + (N/i); } } return maxSum; } int main(){ int N = 18; cout<<"The sum of distinct numbers with LCM as "<<N<<" is "<<calcFactorSum(N); return 0; }
Output
The sum of distinct numbers with LCM as 18 is 39