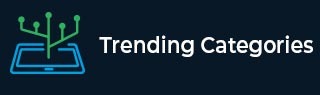
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum sum and product of the M consecutive digits in a number in C++
In this article, we are given a string representing a number. Our task is to create a program in C++ to find the maximum sum and product of M consecutive digits from the given number. We find all sequences of M consecutive digits and return the maximum sum and product.
In mathematics, consecutive numbers are defined as those numbers that follow each other in increasing order from the smallest to the largest, with no missing numbers in between.
This problem can be iterated through the string representation of the number, in other words, we consider consecutive segments of the length of M, carrying out the sum and product of the digits in the segments.
We will always check the highest sum and highest product volume which were met before. If we undergo cyclic repetition through the entire string, we'll get a maximum sum and a maximum product of M consecutive digits.
Maximum Sum of M Consecutive Digits: Consequently, the parties should expend their utmost effort and if possible, look through the whole range of numbers for M consecutive digits whose sum is the highest value of all the M-digit sequences.
Maximum Product of M Consecutive Digits: In the same way, it finds the maximum M-digit combinations by identifying those M digits within the number, whose products are the largest.
Let's go through the input and output scenario to understand how input values relate to the desired output −
Input
number = 2379641, M = 4
Output
maxSum = 26 maxProd = 1512
Explanation
These are all the given subsequences of size 4: 2379, 3796, 7964, and 9641.
The maximum sum = 7 + 9 + 6 + 4 = 26
The maximum product = 7 * 9 * 6 * 4 = 1512
Solution Approach
A simple solution to the problem is to find all possible consecutive subsequences of size M from the number, and then add and multiply all values of the integer and return the maximum of all sum and product values.
Example
Following is the program to find the Maximum sum and product of the M consecutive digits in a number in C++ −
#include <iostream> using namespace std; int findMaxVal(int x, int y){ if(x > y) return x; return y; } void calcMaxProductAndSum(string number, int M){ int N = number.length(); int maxProd = -1, maxSum = -1; int product = 1; int sum = 0; for (int i = 0; i < N - M; i++){ product = 1, sum = 0; for (int j = i; j < M + i; j++){ product = product * (number[j] - '0'); sum = sum + (number[j] - '0'); } maxProd = findMaxVal(maxProd, product); maxSum = findMaxVal(maxSum, sum); } cout<<"The maximum product of "<<M<<" consecutive digits in number "<<number<<" is: "<<maxProd<<endl; cout<<"The sum of "<<M<<" consecutive digits in number "<<number<<" is: "<<maxSum; } int main() { string str = "2379641"; int m = 4; calcMaxProductAndSum(str, m); }
Output
The maximum product of 4 consecutive digits in number 2379641 is: 1512 The sum of 4 consecutive digits in number 2379641 is: 26