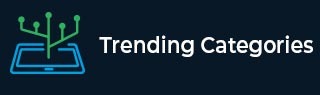
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum subarray sum modulo m in C++
In this problem, we are given an array of size n and an integer m. our task is to create a program that will find the maximum subarray sum modulo m in C++.
Program description − Here, we will find the maximum value obtained by dividing the sum of all elements of subarray divided by m.
Let’s take an example to understand the problem,
Input − array = {4, 9 ,2} m = 6
Output − 5
Explanation − All subarrays and their remainders on dividing
{4}: 4%6 = 4 {9}: 9%6 = 3 {2}: 2%6 = 2 {4, 9}: 13%6 = 1 {9, 2}: 11%6 = 5 {4, 9, 2}: 15%6 = 3
To solve this problem, we compute prefixSumModulo Array. And the calculating maxSum of each index using the formula, maxSum at i = (prefixi + prefixj + m)%m
Example
Program to illustrate our solution,
#include<bits/stdc++.h> using namespace std; int calcMaxSum(int arr[], int n, int m) { int x, prefix = 0, maxSumMod = 0; set<int> sums; sums.insert(0); for (int i = 0; i < n; i++){ prefix = (prefix + arr[i])%m; maxSumMod = max(maxSumMod, prefix); auto it = sums.lower_bound(prefix+1); if (it != sums.end()) maxSumMod = max(maxSumMod, prefix - (*it) + m ); sums.insert(prefix); } return maxSumMod; } int main() { int arr[] = {4, 9, 2}; int n = sizeof(arr)/sizeof(arr[0]); int m = 5; cout<<"Maximum subarray sum modulo "<<m<<" is "<<calcMaxSum(arr, n, m) < endl; return 0; }
Output
Maximum subarray sum modulo 5 is 4
Advertisements