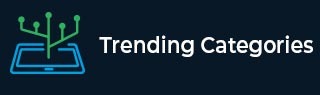
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum profit after buying and selling the stocks in C++
In this problem, we are given an array stkprice[] that denotes the price of a certain stock on i-th day. Our task is to create a program to calculate Maximum profit after buying and selling the stocks in C++.
Problem Description − Here, we need to check when can be bought and sell the stock to gain profit. To gain profit, we need to buy the stock at a low price and sell it when the price goes up. And repeat the same when the drop is encountered again.
Let’s take an example to understand the problem,
Input
stkprice[] = {120, 310, 405, 210, 150, 550}
Output
685
Explanation
Buying on day 1 and selling on day 3, will give a profit of 285.
After this, Buying on day 5 and selling on day 6, will give a profit of 400.
This makes total profit of (400 + 285) = 685
Solution Approach
A simple solution will be checking all possible combinations of the buy-sell cycle. Try buying-selling cycle combinations from every day to buying on first selling on last for the current day. And taking the cycle that yields that maximum profit.
Program to illustrate the working of our solution,
Example
#include <iostream> using namespace std; int max(int a, int b){ if(a > b) return a; return b; } int MaximizeProfit(int stkPrice[], int firstDay, int lastDay){ if (lastDay <= firstDay) return 0; int maxProfit = 0; for (int i = firstDay; i < lastDay; i++) { for (int j = i + 1; j <= lastDay; j++) { if (stkPrice[j] > stkPrice[i]) { int profit = ( stkPrice[j] - stkPrice[i] ) + MaximizeProfit(stkPrice, firstDay, i - 1) + MaximizeProfit(stkPrice, j + 1, lastDay); maxProfit = max(maxProfit, profit); } } } return maxProfit; } int main(){ int stkPrice[] = { 120, 310, 405, 210, 150, 550 }; int days = 6 ; cout<<"The Maximum profit is "<<MaximizeProfit(stkPrice, 0, days); return 0; }
Output
The Maximum profit is 4196007
A more effective solution is based on finding the maximum profit from them by finding the maximum profit for each trade. This can be done by finding local minima and maxima for the trade days. Local minima are those days where the stock price is less than both its previous and next day. And maxima is opposite to the minima. If there are no local minima (within index 0 to n-2), their days cannot be profitable.
To maximize the profit, we will buy the share at local minima and sell it at the next local maxima and do the same for the minima-maxima pair. Adding all the minima-maxima profits we will get the maxProfit.
Program to illustrate the working of our solution,
Example
#include <iostream> using namespace std; void MaximizeProfit(int price[], int n){ if (n == 1) return; int maxProfit = 0; int i = 0; while (i <= n - 1) { while ((i <= n - 2) && (price[i + 1] <= price[i])) i++; int minima = i++; while ((i < n) && (price[i] >= price[i - 1])) i++; int maxima = i - 1; maxProfit += (price[maxima] - price[minima] ); // For displaying profit for one minima-maxima cycle //cout <<"Stock bought on day "<<(minima+ 1 )<<" and Sold on day "<<(maxima+1) <<" at a profit of "<<(price[maxima] - price[minima] )<<"\n"; } cout<<"The maximized profit is "<<maxProfit; } int main(){ int stkPrice[] = { 120, 310, 405, 210, 150, 550 }; int days = 6; MaximizeProfit(stkPrice, days); return 0; }
Output
The maximized profit is 685