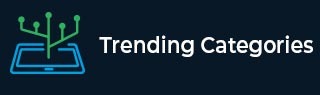
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum of Absolute Value Expression in C++
Suppose we have two arrays of integers with equal lengths, we have to find the maximum value of: |arr1[i] - arr1[j]| + |arr2[i] - arr2[j]| + |i - j|. Where the maximum value is taken over all 0 <= i, j < arr1.length. So if the given two arrays are [1,2,3,4] and [-1,4,5,6], the output will be 13.
To solve this, we will follow these steps −
Define a method called getVal, that will take array v
maxVal := -inf, minVal := inf
for i in range 0 to size of v
minVal := min of v[i] and minVal
maxVal := max of v[i] and maxVal
return maxVal – minVal
From the main method, do the following
make an array ret of size 4
n := size of arr1
for i in range 0 to n – 1
insert arr1[i] – arr2[i] + i into ret[0]
insert arr1[i] + arr2[i] + i into ret[1]
insert arr1[i] – arr2[i] - i into ret[2]
insert arr1[i] + arr2[i] - i into ret[3]
ans := -inf
for i in range 0 to 3
ans := max of ans and getVal(ret[i])
return ans
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: int getVal(vector <int>& v){ int maxVal = INT_MIN; int minVal = INT_MAX; for(int i = 0; i < v.size(); i++){ minVal = min(v[i], minVal); maxVal = max(v[i], maxVal); } return maxVal - minVal; } int maxAbsValExpr(vector<int>& arr1, vector<int>& arr2) { vector <int> ret[4]; int n = arr1.size(); for(int i = 0; i < n; i++){ ret[0].push_back(arr1[i] - arr2[i] + i); ret[1].push_back(arr1[i] + arr2[i] + i); ret[2].push_back(arr1[i] - arr2[i] - i); ret[3].push_back(arr1[i] + arr2[i] - i); } int ans = INT_MIN; for(int i = 0; i < 4; i++){ ans = max(ans, getVal(ret[i])); } return ans; } }; main(){ vector<int> v1 = {1,2,3,4}, v2 = {-1, 4, 5, 6}; Solution ob; cout << (ob.maxAbsValExpr(v1, v2)); }
Input
[1,2,3,4] [-1,4,5,6]
Output
13