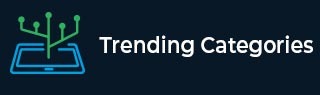
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum no. of contiguous Prime Numbers in an array in C++
We are given with an array of prime numbers, arranged in random order. The size of the array is N. The goal is to find the longest sequence of contiguous prime numbers in the array.
Prime number is the one which has only two factors, 1 and the number itself. 1,2,3,5,7,11,13….are prime numbers whereas 4,6,8,9,10….20 are non-prime. Every non-prime number has more than 2 factors. Let’s understand with examples.
Input − Arr[] = { 1,3,5,2,6,7,13,4,9,10
Output − 3
Explanation − The prime numbers in the above array are 3,5,2,7,13. The numbers that are contiguous are 3,5,2 and 7,13. Longest sequence has 3 numbers. So the answer is 4.
Input − Arr[] = { 5,7,17,27,31,21,41 }.
Output − 3
Explanation − The prime numbers in the above array are 5,7,17,31,41. The numbers that are contiguous are 5,7,17. Longest sequence has 3 numbers. So the answer is 3.
Approach used in the below program is as follows
The integer array Arr[] is used to store the prime and non-prime numbers.
Function isprime(int num) is used to check whether the num is prime or not. If it is prime then it must have no factor till it’s half is reached.
For prime numbers isprime() returns 1 else it returns 0.
Function primeSubarray(int arr[], int n) takes two input parameters. The array of numbers itself, its size. Returns the size of the longest sequence of contiguous prime numbers.
Traverse the numbers in arr[] from start. If arr[i] is non-prime ( isprime(arr[i])==0 ). Then change the count of contiguous prime numbers as 0.
If it is prime, then increment the count of prime numbers. (It will start from 0 again if nonprime is encountered)
Check if the count is maximum so far, if yes store it’s value in maxC.
Return the maxC as result.
Example
#include <iostream> #include <stdio.h> int isprime(int num){ if (num <= 1) return 0; for (int i = 2; i <= num/2; i++) if (num % i == 0) return 0; return 1; //if both failed then num is prime } int primeSubarray(int arr[], int n){ int count=0; int maxSeq=0; for (int i = 0; i < n; i++) { // if non-prime if (isprime(arr[i]) == 0) count = 0; //if prime else { count++; //printf("\n%d",arr[i]); print prime number subsequence maxSeq=count>maxSeq?count:maxSeq; } } return maxSeq; } int main(){ int arr[] = { 8,4,2,1,3,5,7,9 }; int n =8; printf("Maximum no. of contiguous Prime Numbers in an array: %d", primeSubarray(arr, n)); return 0; }
Output
If we run the above code it will generate the following output −
Maximum no. of contiguous Prime Numbers in an array : 3