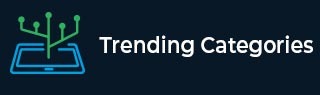
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum difference between two subsets of m elements in C
The task is to find the greatest difference between the sum of m elements in an array. Suppose we have an array and a number m, then we will first find the sum of highest m numbers and then subtract the sum of lowest m numbers from it to get the maximum difference. So the main thing is to find two subsets of m numbers which have the highest sum and lowest sum.
Let’s now understand what we have to do using an example −
Input
arr = {1,2,3,4,5} ; m=3
Output
Maximum difference here is : 6
Explanation − Here the highest 3 numbers are 3,4,5 and the sum is 12. Lowest 3 numbers are 1,2,3 and sum is 6. So the highest or maximum difference is 12-6 which is 6.
Input
arr = {10,13,22,8,16,14}; m=4
Output
Maximum difference here is : 20
Explanation − Here the highest 4 numbers are 22,16,14,13 and the sum is 65. Lowest 4 numbers are 8,10,13,14 and the sum is 45 . So the highest or maximum difference is 65-45 which is 20.
Approach used in the below program as follows
Take input array arr[] and a number m for making sets
In the find_diff() function we are passing the input array and it’s length and returning the maximum difference of the sum of sets of m elements.
Here we will first sort the elements of array arr[].
Then we will find the sum of first m and last m elements as these will be least m and highest m numbers of arr[] .
At last we return the difference.
Note − sort(arr[],int) is assumed to return the sorted array.
Example
#include<stdio.h> //create function to calculate maximum difference between sum of highest and lowest m elements of array int find_diff(int arr[],int length,int m){ //for sorting the array sort(arr,length); int maxsum=0, minsum=0; //calculate now max difference between two subsets of m elements for(int i=0;i<m;i++){ minsum+=arr[i]; maxsum+=arr[length-i-1]; } return(maxsum-minsum); } // Driver program int main(){ int arr[]={1,1,2,3,5,7,1}; int m=3; cout << find_diff(arr,7,m); return 0; }
Output
If we run the above code we will get the following output −
12