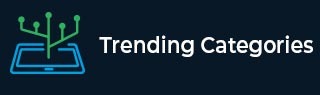
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximum circular subarray sum in C++
We are given an array and the task is to form the subarrays such that the sum of subarrays in a circular fashion will result in a maximum value.
Input − int arr[] = {1, 2, 8, 4, 3, 0, 7}
Output − Maximum circular subarray sum is − 22
Explanation − we are given an array containing {1, 2, 8, 4, 3, 0, 7} and the subarray of it with maximum sum will be 7 + 1 + 2+ 8 + 4 is 22.
Input − int arr[] = { 2, 5, -1, 6, 9, 4, -5 }
Output − Maximum circular subarray sum is − 25
Explanation − we are given an array containing {2, 5, -1, 6, 9, 4, -5} and the subarray of it with maximum sum will be 4 + 2 + 5 - 1 + 6 + 9 is 25.
Approach used in the below program is as follows
Input an array of integer elements containing both positive and negative values.
Calculate the size of an array.
Pass an array and the size to the function for further processing.
Create a temporary variable as total and set it to 0
Start loop FOR from i to 0 till the size of an array
Inside the loop, Set total with total + arr[i]
Set temp = arr[0], temp_2 = arr[0], temp_3 = arr[0], temp_4 = arr[0]
Start the loop FOR from i to 1 till the size of an array
Inside the loop set temp = max(temp + arr[i], arr[i]), temp_2 = max(temp_2, temp), temp_3 = min(temp_3 + arr[i], arr[i]), temp_4 = min(temp_4, temp_3)
Check IF size == 1 then return arr[0]
Check IF temp_4 == total then return temp_2
Set max_sum = max(temp_3, total - temp_4)
Return max_sum
Print the result.
Example
#include <bits/stdc++.h> using namespace std; int maximum(int arr[], int size){ int total = 0; for (int i = 0; i < size; i++){ total += arr[i]; } int temp = arr[0]; int temp_2 = arr[0]; int temp_3 = arr[0]; int temp_4 = arr[0]; for (int i = 1; i < size; i++){ temp = max(temp + arr[i], arr[i]); temp_2 = max(temp_2, temp); temp_3 = min(temp_3 + arr[i], arr[i]); temp_4 = min(temp_4, temp_3); } if (size == 1){ return arr[0]; } if (temp_4 == total){ return temp_2; } int max_sum = max(temp_3, total - temp_4); return max_sum; } int main(){ int arr[] = { 2, 5, -1, 6, 9, 4, -5 }; int size = sizeof(arr) / sizeof(arr[0]); cout<<"Maximum circular subarray sum is: "<<maximum(arr, size) << endl; return 0; }
Output
If we run the above code it will generate the following output −
Maximum circular subarray sum is: 25