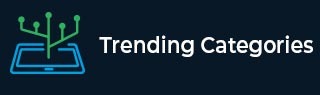
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximize volume of cuboid with given sum of sides in C++
We are given with a sum of sides of a cuboid. A cuboid has three sides length, breadth and height. The volume of cuboid is calculated as a product of all three sides.
Volume of Cuboid = Length X Breadth X Height
The maximum volume can be achieved if all three sides are as close as possible.
Let’s now understand what we have to do using an example −
For Example
The problem given here provides us with the sum of sides, say S. And let sides be L, B, H. In order to maximize the volume we have to find the sides as close as possible. Let’s say we have S=6. Possible sides could be −
[L=1,B=1,H=4] volume=4 [L=1,B=2,H=3] volume=6 [L=2,B=2,H=2] volume=8
Note − other combinations will have the same results. So maximum volume is achieved when L,B,H are close or equal to each other.
Therefore −
Input − S=6
Output − Maximized volume of the cuboid with given sum of sides is 8.
Explanation − Let’s divide sum S into L,B,H equally as much as possible.
L=S/3 ----> (L=2 integer part, remaining S is 4) B=(S-L)/2=(S-S/3)/2 ----> (B=2, remaining S is 2) H=S-L-B = S-S/3-(S-S/3) ----> (H=2, remaining S is 0)
Input − S=10
Output − The maximum volume of the cuboid with a given sum of sides is 36.
Explanation − Let’s divide sum S into L,B,H equally as much as possible.
L=S/3 ----> (L=3 integer part, remaining S is 7) B=(S-L)/2=(S-S/3)/2 ----> (B=3, remaining S is 4) H=S-L-B = S-S/3-(S-S/3) ----> (H=4, remaining S is 0)
Approach used in the below program as follows
Take input as Sum from the user.
Calculate Length as Sum/3 (Integer arithmetic) and update Sum as Sum-Length.
Calculate Breadth as Sum/2 (Integer arithmetic) and update Sum as Sum-Breadth.
Now assign the remaining Sum to Height.
Note − The order of calculation for sides does not matter.
Example
#include <bits/stdc++.h> using namespace std; int Maximize_Volume(int sumofsides){ int length,breadth,height; length=breadth=height=0; // finding length length = sumofsides / 3; sumofsides -= length; // finding breadth breadth = sumofsides / 2; // remaining sumofsides is height height = sumofsides - breadth; return length * breadth * height; } // Driven Program int main(){ int sos = 12; cout << "Maximized volume of the cuboid with given sum of sides is "<<Maximize_Volume(sos) << endl; return 0; }
Output
If we run the above code we will get the following output −
Maximized volume of the cuboid with given sum of sides is 64