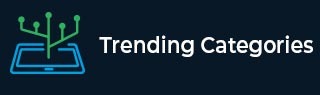
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximize the number by rearranging bits in C++
Problem statement
Given an unsigned number, find the maximum number that could be formed by using the bits of the given unsigned number
If the input number is 8 then its binary representation is−
00000000000000000000000000001000
To maximize it set MSB to 1. Then number becomes 2147483648 whose binary representation is−
10000000000000000000000000000000
Algorithms
1. Count number of set bits in the binary representation of a given number 2. Find a number with n least significant set bits 3. shift the number left by (32 – n)
Example
#include <bits/stdc++.h> using namespace std; unsigned getMaxNumber(unsigned num){ int n = __builtin_popcount(num); if (n == 32) { return num; } unsigned result = (1 << n) - 1; return (result << (32 - n)); } int main(){ unsigned n = 8; cout << "Maximum number = " << getMaxNumber(n) << endl; return 0; }
Output
When you compile and execute the above program. It generates the following output−
Maximum number = 2147483648
Advertisements