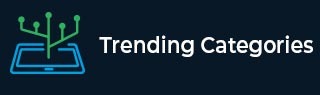
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximize the given number by replacing a segment of digits with the alternate digits given in C++
Given the task is to maximize a given number with ‘N’ number of digits by replacing its digit using another array that contains 10 digits as an alternative for all single-digit numbers 0 to 9,
The given condition is that only a consecutive segment of numbers can be replaced and only once.
Input
N=1234, arr[]={3 ,0 ,1 ,5 ,7 ,7 ,8 ,2 ,9 ,4}
Output
1257
Explanation
The number 3 can be replaced with its alternative 5= arr[3]
The number 4 can be replaced with its alternative 7= arr[4]
Input
N=5183, arr[]={3 ,0 ,1 ,5 ,7 ,7 ,8 ,2 ,9 ,4}
Output
7183
Approach used in the below program as follows
In function Max() create a variable ‘N’ of type int to store the size of the number.
Loop from i=0 till i<N and check for the number whose alternative is greater than it.
Then replace the number with its alternative.
Do this for the upcoming number until a number having a smaller alternative is not found
Example
#include <bits/stdc++.h> using namespace std; string Max(string str, int arr[]){ int N = str.size(); //Iterating till the end of string for (int i = 0; i < N; i++) { //Checking if it is greater or not if (str[i] - '0' < arr[str[i] - '0']) { int j = i; //Replacing with the alternate till smaller while (j < N && (str[j] - '0' <= arr[str[j] - '0'])) { str[j] = '0' + arr[str[j] - '0']; j++; } return str; } } // Returning original str if there is no change return str; } //Main function int main(){ string str = "2075"; int arr[] = {3 ,0 ,1 ,5 ,7 ,7 ,8 ,2 ,9 ,4 }; cout <<” Maximize the given number by replacing a segment of digits with the alternate digits given is: ” <<Max(str, arr); return 0; }
Output
If we run the above code we will get the following output −
Maximize the given number by replacing a segment of digits with the alternate digits given is: 2375