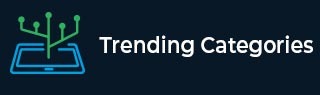
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Maximize Sum Of Array After K Negations in Python
Suppose we have an array A of integers, we have to modify the array in the following way −
We can choose an i and replace the A[i] with -A[i], and we will repeat this process K times. We have to return the largest possible sum of the array after changing it in this way.
So, if the array A = [4,2,3], and K = 1, then the output will be 5. So choose indices 1, the array will become [4,-2,3]
To solve this, we will follow these steps −
Sort the array A
for i in range 0 to length of A – 1
if A[i] <0, then A[i] := - A[i], and decrease k by 1
if k = 0, then break from the loop
if k is even, then
sp := A[0]
for i := 1 to length of A – 1
if A[i] > 0, then sp := minimum of sp and A[i]
return sum of the elements of A – (2*sp)
otherwise, return the sum of the elements of A
Example (Python)
Let us see the following implementation to get a better understanding −
class Solution(object): def largestSumAfterKNegations(self, A, K): A.sort() for i in range(len(A)): if A[i] <0: A[i] = -A[i] K-=1 if K==0: break if K%2: smallest_positive = A[0] for i in range(1,len(A)): if A[i]>=0: smallest_positive = min(smallest_positive,A[i]) return sum(A) - (2*smallest_positive) else: return sum(A) ob1 = Solution() print(ob1.largestSumAfterKNegations([3,-1,0,2],3))
Input
[3,-1,0,2] 3
Output
6