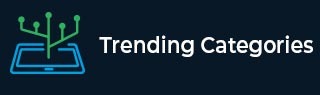
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Matching a whole word Java Regular expressions:
The meta character "\b" matches word boundaries. i.e. it matches before the first and after the last word characters and between word and non-word characters.
Therefore to match a whole word you need to surround it between the word boundary meta characters as −
\btest\b
Example
Following Java example counts and prints the number of occurrences of the word test in the given input string.
import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample1 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter input text: "); String input = sc.nextLine(); String regex = "\btest\b"; //Creating a pattern object Pattern pattern = Pattern.compile(regex); //Matching the compiled pattern in the String Matcher matcher = pattern.matcher(input); int count =0; while (matcher.find()) { count++; } System.out.println("Number of occurrences of the word test : "+count); } }
Output
Enter input text: sample data: test test test Number of occurrences of the word test : 3
Advertisements