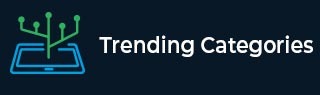
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Make an array of another array's duplicate values in JavaScript
In the given problem statement we are asked to make an array of another array’s duplicate values with the help of javascript functionalities. As we talk about duplicate values in an array means we have to find the exact elements present in the first array.
What is an array in JavaScript ?
Let's understand the working of an array in JavaScript.
An array is an object that stores multiple elements. In programming, an array is a collection of similar data elements under one roof. Since an array is also an object, it has some properties and methods that work with arrays easier in JavaScript. On arrays we can perform filter() and indexOf() methods.
Following is the syntax to define list in JavaScript : −
Array = [ 201, 202, 405 ]
Understanding The Logic
To define an array of duplicate arrays with the same items in javascript, we can use a combination of the filter() and indexOf() array methods. We will have two arrays arr1 and arr2. Now we have to find the values that are present in both the arrays. So we will use the filter() method on arr1 and check if the value present in arr2 with the help of indexOf() method. Then the indexOf() method will return a value other than −1, this means the value is present in arr2. And we will add it to the duplicates array.
In the last, the identical array will contain all the elements between arr1 and arr2.
Algorithm
Step 1 : Declare a function named itemSearch taking in a list and the element to search as arguments.
Step 2 : Declare the left and right pointer variables to find the exact location of the search element.
Step 3 : The function we have declared just above in Step 1 is basically a recursive function. The function is working with a while loop inside it. This loop will run until the left element is less than or equal to the right element.
Step 4 : Now forwarding , after the while loop we will calculate the middle element by adding left and right elements and divide the sum by 2. So in this step we have our mid element.
Step 5 : The following Step 4 needs to be recursively done for that particular mid element. Next we will check that the search item is less or greater than the mid item. If it is less than a search term then find it in the left side of the list otherwise search in the right side of the mid element.
Step 6 : Now for checking the search term we will use the middle variable to check the conditions with the mid element. If middleElement is less than the search item then increase the middle count by 1. Otherwise decrease the middle count by 1.
Step 7 : This is how the recursive binary search will work. Now in this step define a sorted list and search item and call the function to get the output.
Example
// define two array for duplicates const arr1 = [11, 12, 13, 14, 15, 16, 17, 18, 19]; const arr2 = [13, 16, 19, 2]; // define an object to find duplicates const duplicates = arr1.filter((value) => { return arr2.indexOf(value) !== -1; }); // show output console.log(duplicates);
Output
[ 13, 16, 19 ]
The code given above is the most straightforward one that comes to mind on reading the issue statement, but if you grasp the theory behind it, you can simplify and adapt it to make the most efficient use of time and space.
Two arrays named arr1 and arr2 with some similar values each have been declared in the code above. Finding the duplicates and making another array of those elements is our responsibility.
Consequently, we will utilize the "filter" and "indexOf" methods to create an array of duplicates.
Time Complexity
The amount of time needed to execute a specific function or group of statements is measured by an algorithm. Additionally, it is based on the size of the function's inputs. The time the algorithm requires in our situation is O(n), where n is the number of entries in the array.
And space complexity of an algorithm is a measure of memory amount required to store the output. It is generally figured out in terms of the number of memory locations. Those are required to complete the execution.
Conclusion
This is the most easy and reliable method to create an array of duplicates for an element in an array in JavaScript is with the help of a filter() and indexOf() methods. We have come to the conclusion that algorithms are a very important procedure in computer science. In our problem we have seen a simple algorithm to solve the issue and code after thinking logically.