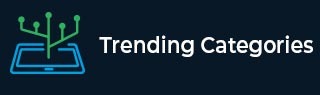
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Loop through an index of an array to search for a certain letter in JavaScript
We are required to write a JavaScript function that takes in an array of strings as the first argument and a single character as the second argument.
The function should return true if the character specified by second argument exists in any of the strings of the array, false otherwise.
Example
The code for this will be −
const arr = ['first', 'second', 'third', 'last']; const searchForLetter = (arr = [], letter = '') => { for(let i = 0; i < arr.length; i++){ const el = arr[i]; if(!el.includes(letter)){ continue; }; return true; }; return false; }; console.log(searchForLetter(arr, 's')); console.log(searchForLetter(arr, 'x'));
Output
And the output in the console will be −
true false
Advertisements