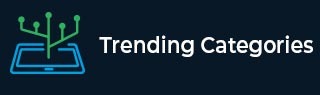
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Longest Harmonious Subsequence in C++
Suppose we have an integer array; we have to find the length of its longest harmonious subsequence among all its possible subsequences. As we know a harmonious sequence array is an array where the difference between its maximum value and its minimum value is exactly 1.
So, if the input is like [1,3,2,2,5,2,3,7], then the output will be 5, as the longest harmonious subsequence is [4,3,3,3,4].
To solve this, we will follow these steps −
Define one map m
for n in nums −
(increase m[n] by 1)
for key-value pair (k,v) in m −
it := position of (k+1) in m
if 'it' is in m, then −
max_:= maximum of max_ and value of it
return max_
Example
Let us see the following implementation to get a better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: int findLHS(vector<int>& nums) { unordered_map<int, int> m; for (const int n : nums) ++m[n]; int max_{ 0 }; for (const auto & [ k, v ] : m) { auto it = m.find(k + 1); if (it != m.end()) max_ = max(max_, v + it->second); } return max_; } }; main(){ Solution ob; vector<int> v = {2,4,3,3,6,3,4,8}; cout << (ob.findLHS(v)); }
Input
{2,4,3,3,6,3,4,8}
Output
5
Advertisements