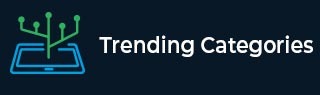
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Longest distance between 1s in binary JavaScript
We are required to write a JavaScript function that in a positive integer, say n. The function should find and return the longest distance between any two adjacent 1's in the binary representation of n.
If there are no two adjacent 1's, then we have to return 0.
Two 1's are adjacent if there are only 0's separating them (possibly no 0's). The distance between two 1's is the absolute difference between their bit positions. For example, the two 1's in "1001" have a distance of 3.
For example −
If the input is 22, then the output should be 2,
because,
- The binary code for 22 is 10110
- The first adjacent pair of 1's is "10110" with a distance of 2.
- The second adjacent pair of 1's is "10110" with a distance of 1.
- The answer is the largest of these two distances, which is 2.
Note that "10110" is not a valid pair since there is a 1 separating the two 1's underlined.
Example
const num = 22; const binaryGap = (num = 1) => { let last = -1; let ans = 0; // go through every bit for (let i = 0; i < 32; i++) { // check whether the bit is `1`. // if true, calculate the longest distance with // previous `1` if `1` was previously found. if ((num >> i) & 1 > 0) { if (last >= 0) { ans = Math.max(ans, i - last); } last = i; } } return ans; }; console.log(binaryGap(num));
Output
And the output in the console will be −
2
Advertisements