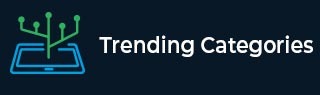
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
logical_and in C++
In this article, we will be discussing the working, syntax, and examples of logical_and function object class in C++.
What is logical_and?
logical_and binary function is an inbuilt binary function object class in C++, which is defined in a <functional> header file. logical_and is a binary function used to give the result of logical “and” operation between two arguments.
Logical AND is the binary operation that returns true only and only if both the binary values are true.
Syntax of logical_and
Template struct logical_and : binary_function { T operator() (const T& a, const T& b) const {return a&b&; } };
Template parameters
The function accepts the following parameter(s) −
T − This is the type of the argument passed to function call.
Example
#include <bits/stdc++.h> using namespace std; int main(){ bool a[] = { true, false, true, false, true }; bool b[] = { true, true, false, false, true }; int ele = 5; bool output[ele]; transform(a, a + ele, b, output, logical_and<bool>()); cout<<"The result for Logical AND is: \n"; for (int i = 0; i < ele; i++){ cout << a[i] << " AND " << b[i] << " is: " <<output[i] << "\n"; } return 0; }
Output
If we run the above code it will generate the following output −
The result for Logical AND is: 1 AND 1 is: 1 0 AND 1 is: 0 1 AND 0 is: 0 0 AND 0 is: 0 1 AND 1 is: 1
Advertisements