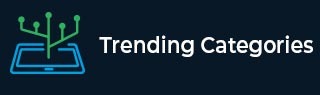
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
log() function for complex number in C++
In this article we will be discussing the working, syntax and examples of log() function in C++ STL.
What is the log() function?
log() function is an inbuilt function in C++ STL, which is defined in <complex> header file. log() returns complex natural logarithmic value of a complex value. The difference between the log() in math header file and log() of complex header file is that it is used to calculate the complex logarithmic where log() of math header file calculates normal logarithmic value.
Syntax
template<class T> complex<T> log(const complex<T>& x);
Parameters
This function accepts one parameter which is a complex value whose log we have to find.
Return value
The logarithmic value of x we want to calculate.
Example
Input: complex<double> C_number(-7.0, 1.0); log(C_number); Output: log of (-7,1) is (1.95601,2.9997)
#include <bits/stdc++.h> using namespace std; int main() { complex<double> C_number(-7.0, 1.0); cout<<"log of "<<C_number<<" is "<<log(C_number)<< endl; return 0; }
Output
If we run the above code it will generate the following output −
log of (-7,1) is (1.95601,2.9997)
Example
#include <bits/stdc++.h> using namespace std; int main() { complex<double> C_number(-4.0, -1.0); cout<<"log of "<< C_number<< " is "<<log(C_number); return 0; }
Output
If we run the above code it will generate the following output −
log of (-4,-1) is (1.41661,-2.89661)
Advertisements