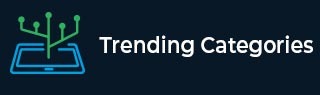
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
ListDictionary Class in C#
The ListDictionary class implements IDictionary using a singly linked list. It is recommended for collections that typically include fewer than 10 items.
Following are the properties of ListDictionary class −
Sr.No | Property & Description |
---|---|
1 | Count Gets the number of key/value pairs contained in the ListDictionary. |
2 | IsFixedSize Gets a value indicating whether the ListDictionary has a fixed size. |
3 | IsReadOnly Gets a value indicating whether the ListDictionary is readonly. |
4 | IsSynchronized Gets a value indicating whether the ListDictionary is synchronized (thread safe). |
5 | Item[Object] Gets or sets the value associated with the specified. |
6 | Keys Gets an ICollection containing the keys in the ListDictionary. |
7 | SyncRoot Gets an object that can be used to synchronize access to the ListDictionary. |
8 | Values Gets an ICollection containing the values in the ListDictionary. |
Following are some of the methods of the ListDictionary class −
Sr.No | Methods & Description |
---|---|
1 | Add(Object, Object) Adds an entry with the specified key and value into the ListDictionary. |
2 | Clear() Removes all entries from the ListDictionary. |
3 | Contains(Object) Determines whether the ListDictionary contains a specific key. |
4 | CopyTo(Array, Int32) Copies the ListDictionary entries to a onedimensional Array instance at the specified index. |
5 | Equals(Object) Determines whether the specified object is equal to the current object. (Inherited from Object) |
6 | GetEnumerator() Returns an IDictionaryEnumerator that iterates through the ListDictionary. |
7 | GetHashCode() Serves as the default hash function. (Inherited from Object) |
8 | GetType() Gets the Type of the current instance. (Inherited from Object) |
Example
Let us now see some examples −
To check if ListDictionary is read-only, the code is as follows −
using System; using System.Collections; using System.Collections.Specialized; public class Demo { public static void Main() { ListDictionary dict1 = new ListDictionary(); dict1.Add("A", "Books"); dict1.Add("B", "Electronics"); dict1.Add("C", "Smart Wearables"); dict1.Add("D", "Pet Supplies"); dict1.Add("E", "Clothing"); dict1.Add("F", "Footwear"); Console.WriteLine("ListDictionary1 elements..."); foreach(DictionaryEntry d in dict1) { Console.WriteLine(d.Key + " " + d.Value); } Console.WriteLine("Is the ListDictionary1 having fixed size? = "+dict1.IsFixedSize); Console.WriteLine("If ListDictionary1 read-only? = "+dict1.IsReadOnly); Console.WriteLine("Is ListDictionary1 synchronized = "+dict1.IsSynchronized); Console.WriteLine("The ListDictionary1 has the key M? = "+dict1.Contains("M")); ListDictionary dict2 = new ListDictionary(); dict2.Add("1", "One"); dict2.Add("2", "Two"); dict2.Add("3", "Three"); dict2.Add("4", "Four"); dict2.Add("5", "Five"); dict2.Add("6", "Six"); Console.WriteLine("
ListDictionary2 key-value pairs..."); IDictionaryEnumerator demoEnum = dict2.GetEnumerator(); while (demoEnum.MoveNext()) Console.WriteLine("Key = " + demoEnum.Key + ", Value = "+ demoEnum.Value); Console.WriteLine("Is the ListDictionary2 having fixed size? = "+dict2.IsFixedSize); Console.WriteLine("If ListDictionary2 read-only? = "+dict2.IsReadOnly); Console.WriteLine("Is ListDictionary2 synchronized = "+dict2.IsSynchronized); Console.WriteLine("The ListDictionary2 has the key 5? = "+dict2.Contains("5")); } }
Output
This will produce the following output −
ListDictionary1 elements... A Books B Electronics C Smart Wearables D Pet Supplies E Clothing F Footwear Is the ListDictionary1 having fixed size? = False If ListDictionary1 read-only? = False Is ListDictionary1 synchronized = False The ListDictionary1 has the key M? = False ListDictionary2 key-value pairs... Key = 1, Value = One Key = 2, Value = Two Key = 3, Value = Three Key = 4, Value = Four Key = 5, Value = Five Key = 6, Value = Six Is the ListDictionary2 having fixed size? = False If ListDictionary2 read-only? = False Is ListDictionary2 synchronized = False The ListDictionary2 has the key 5? = True
To check if two ListDictionary objects are equal, the code is as follows −
Example
using System; using System.Collections; using System.Collections.Specialized; public class Demo { public static void Main() { ListDictionary dict1 = new ListDictionary(); dict1.Add("A", "Books"); dict1.Add("B", "Electronics"); dict1.Add("C", "Smart Wearables"); dict1.Add("D", "Pet Supplies"); dict1.Add("E", "Clothing"); dict1.Add("F", "Footwear"); Console.WriteLine("ListDictionary1 elements..."); foreach(DictionaryEntry d in dict1) { Console.WriteLine(d.Key + " " + d.Value); } ListDictionary dict2 = new ListDictionary(); dict2.Add("1", "One"); dict2.Add("2", "Two"); dict2.Add("3", "Three"); dict2.Add("4", "Four"); dict2.Add("5", "Five"); dict2.Add("6", "Six"); Console.WriteLine("
ListDictionary2 elements..."); foreach(DictionaryEntry d in dict2) { Console.WriteLine(d.Key + " " + d.Value); } ListDictionary dict3 = new ListDictionary(); dict3 = dict2; Console.WriteLine("
Is ListDictionary3 equal to ListDictionary2? = "+(dict3.Equals(dict2))); } }
Output
This will produce the following output −
ListDictionary1 elements... A Books B Electronics C Smart Wearables D Pet Supplies E Clothing F Footwear ListDictionary2 elements... 1 One 2 Two 3 Three 4 Four 5 Five 6 Six Is ListDictionary3 equal to ListDictionary2? = True
Advertisements