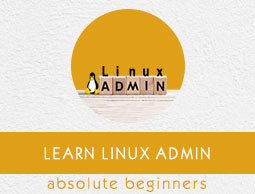
- Linux Admin Tutorial
- Home
- CentOS Overview
- Basic CentOS Linux Commands
- File / Folder Management
- User Management
- Quota Management
- Systemd Services Start and Stop
- Resource Mgmt with systemctl
- Resource Mgmt with crgoups
- Process Management
- Firewall Setup
- Configure PHP in CentOS Linux
- Set Up Python with CentOS Linux
- Configure Ruby on CentOS Linux
- Set Up Perl for CentOS Linux
- Install and Configure Open LDAP
- Create SSL Certificates
- Install Apache Web Server CentOS 7
- MySQL Setup On CentOS 7
- Set Up Postfix MTA and IMAP/POP3
- Install Anonymous FTP
- Remote Management
- Traffic Monitoring in CentOS
- Log Management
- Backup and Recovery
- System Updates
- Shell Scripting
- Package Management
- Volume Management
- Linux Admin Useful Resources
- Linux Admin - Quick Guide
- Linux Admin - Useful Resources
- Linux Admin - Discussion
Linux Admin - Conditionals
While loops are the main control structures for operational flow, logical operations need to be performed as well.
Logical operations can be controlled with the following constructs in BASH: if, then, else, and elif.
If
This is pretty simple and will make a conditional operation based on how a logic test evaluates.
#!/bin/bash result = 1 if [ $result -eq 1 ]; then echo "Result was true!" else echo "Result was false!" fi
Note − Bash uses a few different equality operators. In this case, we used "-eq", performing equality on an integer. For a string, we'd use "==".
elif is used to pass logic to another conditional branch, shown as follows −
#!/bin/bash ourColor="red" if [ $ourColor == "black" ]; then echo "Too dark" elif [ $ourColor == "white" ]; then echo "Too plain!" elif [ $ourColor != "gray" ]; then echo "Too colorful" else echo "Let's make it" $ourColor fi
Our somewhat biased script will not be satisfied until we decide on gray.
linux_admin_shell_scripting.htm
Advertisements