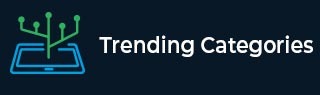
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
linecache.getline() in Python
Learning how to handle files effectively is essential for mastering Python or any other programming language. The Python language has the linecache module as a useful tool. It is an assistance module that makes it possible to read any line from any file while taking care of the technical aspects like caching, file I/O, and error handling. The linecache.getline() function, which can be a great tool in your Python programming toolbox, will be thoroughly examined in this article.
Introduction to Linecache.getline()
To extract a single line of text from a file in Python, use the linecache.getline() function. The caching feature of this function is a huge benefit. The function keeps files that have already been read in memory, making it possible to read succeeding lines more quickly. When dealing with huge files, this capability is quite useful.
An easy function signature is shown below −
linecache.getline(filename, lineno, module_globals=None)
Using Linecache.getline()
Make sure the linecache module is present in your Python environment before continuing. If not, import linecache can be used to import it.
Let's look at few examples of this function.
Example 1: Fetching a Single Line from a File
As an example, have a look at the text file test.txt −
Line 1: This is the first line. Line 2: This is the second line. Line 3: This is the third line. Line 4: This is the fourth line. Line 5: This is the fifth line.
Utilising linecache, you may obtain the third line.getline():
import linecache filename = 'test.txt' print(linecache.getline(filename, 3))
Output
Line 3: This is the third line.
Example 2: Fetching a Line from a Large File
linecache's caching function.Given that it keeps the file in memory after the initial read, getline() is especially helpful when working with huge files. It follows that subsequent readings will be quicker and more effective.
Let's mimic this using the large_file.txt text file.
import linecache import time filename = 'large_file.txt' start_time = time.time() print(linecache.getline(filename, 50000)) # first read print("Time taken for first read: ", time.time() - start_time) start_time = time.time() print(linecache.getline(filename, 100000)) # second read print("Time taken for second read: ", time.time() - start_time)
The results will show how effective linecache is.big files and getline().
Example 3: Error Handling
If you attempt to fetch a queue that doesn't exist, what happens? linecache.Getline graciously processes this and returns an empty string.
import linecache filename = 'test.txt' print(linecache.getline(filename, 1000)) # non-existent line
Output
''
Example 4: Fetching Lines from a Python Script
Python scripts can use the linecache.getline() function as well. Here is an illustration of how to read lines from a Python file −
import linecache filename = 'example.py' # Fetch first line of the Python script print(linecache.getline(filename, 1)) # Fetch fifth line of the Python script print(linecache.getline(filename, 5))
This script pulls the first and fifth lines from the 'example.py' file. Your Python script's content will determine the result.
Example 5: Fetching Lines from Multiple Files
Lines from many files can be efficiently fetched using linecache.getline(). Here's an illustration −
import linecache filenames = ['file1.txt', 'file2.txt', 'file3.txt'] for filename in filenames: print(f'First line in {filename}:') print(linecache.getline(filename, 1))
The first line from each file listed in the 'filenames' list is printed by this script. Keep in mind that the outcome will change depending on what is in these files.
Example 6: Using module_globals Parameter
It is possible to imitate linecache with the module_globals argument.getline() is available for modules loaded with module import *.
import linecache import os filename = 'example.py' # Fetching line from a script with globals print(linecache.getline(filename, 5, globals()))
The global namespace of the active module is passed to module_globals in this example, which retrieves a line from a Python script.
These illustrations highlight linecache.getline()'s adaptability. Linecache.getline() offers a quick way to retrieve lines from text files, Python scripts, or numerous sources.
Conclusion
In summary, linecache.getline() is a crucial Python function for effective file management. It provides a speedy and effective technique to access lines from both small and large files by caching data in memory. By delivering an empty string when an attempt is made to access a line that doesn't exist, it also makes error handling simpler. This article provided an overview of linecache.getline() with practical examples to help you comprehend its advantages.