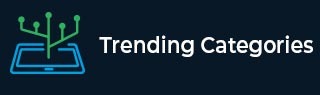
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Lexical Scoping in Dart Programming
Dart is a lexically scoped language. Lexically scoped means that as we move downwards to the latest variable declaration, the variable value will depend on the innermost scope in which the variable is present.
Example
Consider the example shown below −
void main(){ var language = 'Dart'; void printLanguage(){ language = 'DartLang'; print("Language is ${language}"); } printLanguage(); }
In the above example, we changed the value of the language variable inside the scope of the printLanguage() function and since we are printing the value inside the printLanguage() function, the innermost scope is the one inside the same code block, and hence we will get the value of the language = 'DartLang' instead of language = 'Dart'.
Output
Language is DartLang
Example
Let us consider one more example, a bit more complicated one to understand the lexical scoping in a better way.
Consider the example shown below −
void main() { String language = "Dart"; void outerFunction() { String level = 'one'; String ex = "scope"; void innerFunction() { Map level = {'count': "Two"}; print('ex: $ex, level: $level'); print('Language: $language'); } innerFunction(); print('ex: $ex, level: $level'); } outerFunction(); }
There are multiple scopes in the above example, for instance, we have a scope as soon as we start the main function, after that, another inner scope opens as soon as we enter the outerFunction() and an even more scope as we move inside the innerFunction().
Output
ex: scope, level: {count: Two} Language: Dart ex: scope, level: one