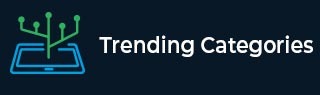
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Legal and illegal declaration and initializations in C
Problem
Mention some of the legal and illegal declarations and initializations while doing C programming?
Before discussing the legal and illegal statements let’s see how to declare and initialize the variables in C.
Variable declaration
Following is the syntax of variable declaration −
Syntax
Datatype v1,v2,… vn;
Where v1, v2,...vn are names of the variables.
For example, int sum;
float a,b;
Variable can be declared in two ways −
local declaration
global declaration
The ‘local declaration’ is declaring a variable inside the main block and its value is available within that block.
The ‘global declaration’ is declaring a variable outside the main block and its value is available throughout the program.
For example,
int a, b; /* global declaration*/ main ( ){ int c; /* local declaration*/ - - - }
Variable initialization
Following is the syntax of variable initialization −
Syntax
Datatype v1=number;
For example,
int sum=0; float a=1,b=4.5;
Declare the variables by using data types, we can initialize the value at the time of declaration. So, while initializing and declaring the values we need to follow the rules
Let’s see some of the examples of legal and illegal declarations and initializations in C.
Example
Char a=65;
It is a legal statement because we can initialize a variable with a constant.
Static int p=20, q=p*p
It is an illegal statement because static variable has to be initialized by a constant but here q is not initialized with a constant.
Double x=30 *PI
It is a legal statement because here we initialized a variable with a constant expression.
Double diameter []={1,PI/2, PI, 2*PI/2}
It is a legal statement, here we initialized array elements with constant.
Sample program
With legal declaration & initialization −
#include<stdio.h> void main ( ){ int a,b; a= 10, b = 20; printf (" %d", a<b); printf (" %d", a<=b); printf (" %d", a>b); printf (" %d", a>=b); printf (" %d", a = =b); printf (" %d", a ! =b); }
Output
1 1 0 0 0 1
Example
With illegal declaration & initialization −
#include <stdio.h> int main(){ static int p=20, q=p*p;//illegal initialization printf("%d%d",p,q); return 0; }
Output
error will be occurred error: initializer element is not constant static int p=20, q=p*p;