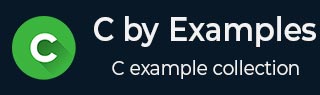
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Variables in C
A variable is a place holder for some value. All variables have some type associated with them, which expresses what 'type' of values they can be assigned. C offers a rich set of variables −
Type | Format String | Description |
---|---|---|
char | %c | Character type variables (ASCII values) |
int | %d | The most natural size of integer for the machine. |
float | %f | A single-precision floating point value. |
double | %e | A double-precision floating point value. |
void | − N/A − | Represents the absence of type. |
Character (char
) variable in C
Character (char
) variable holds a single character.
#include <stdio.h> int main() { char c; // char variable declaration c = 'A'; // defining a char variable printf("value of c is %c", c); return 0; }
The output of the program should be −
value of c is A
Integer (int
) variable in C
int
variable holds singed integer value of single character.
#include <stdio.h> int main() { int i; // integer variable declaration i = 123; // defining integer variable printf("value of i is %d", i); return 0; }
The output of the program should be −
value of i is 123
Floating point (float
) variable in C
float
variable holds single precision floating point values.
#include <stdio.h> int main() { float f; // floating point variable declaration f = 12.001234; // defining float variable printf("value of f is %f", f); return 0; }
The output of the program should be −
value of f is 12.001234
Double precision(double
) floating point variable in C
double
variable holds double precision floating point values.
#include <stdio.h> int main() { double d; // double precision variable declaration d = 12.001234; // defining double precision variable printf("value of d is %e", d); return 0; }
The output of the program should be −
value of d is 1.200123e+01
Void (void
) data type in C
void
in C means "nothing" or "no-value". This is used either with pointer declarations or with function declarations.
// declares function which takes no arguments but returns an integer value int status(void) // declares function which takes an integer value but returns nothing void status(int) // declares a pointer p which points to some unknown type void * p
simple_programs_in_c.htm
Advertisements