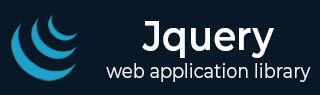
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - slice( start, [end] ) Method
Description
The slice( start, end ) method selects a subset of the matched elements.
Syntax
Here is the simple syntax to use this method −
selector.slice( start, end )
Parameters
Here is the description of all the parameters used by this method −
start − Where to start the subset. The first element is at zero. Can be negative to start from the end of the selection.
end − Where to end the subset excluding end element. If unspecified, ends at the end of the selection.
Example
Following is a simple example a simple showing the usage of this method −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function() { $("li").slice(2, 5).addClass("selected"); }); </script> <style> .selected { color:red; } </style> </head> <body> <div> <ul> <li class = "above">list item 0</li> <li class = "top">list item 1</li> <li class = "top">list item 2</li> <li class = "middle">list item 3</li> <li class = "middle">list item 4</li> <li class = "bottom">list item 5</li> <li class = "bottom">list item 6</li> <li class = "below">list item 7</li> </ul> </div> </body> </html>
This will produce following result −
Example
Following is a simple example a simple showing the usage of this method −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function() { $("li").slice(2, 5).addClass("selected"); }); </script> <style> .selected { color:red; } </style> </head> <body> <div> <ul> <li class = "above">list item 0</li> <li class = "top">list item 1</li> <li class = "selected">list item 2</li> <li class = "selected">list item 3</li> <li class = "selected">list item 4</li> <li class = "bottom">list item 5</li> <li class = "bottom">list item 6</li> <li class = "below">list item 7</li> </ul> </div> </body> </html>
This will produce following result −
jquery-traversing.htm
Advertisements
To Continue Learning Please Login