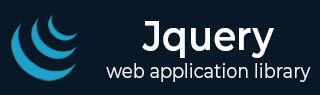
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery prevUntil() Method
The prevUntil() method in jQuery is used to select all preceding siblings elements between the selector and stop. The returned elements will not include the selector and stop.
Note: If no parameters are specified for this method, it will return all next previous elements, that is similar to using the prevAll() method.
Syntax
Following is the syntax of prevUntil() method in jQuery −
$(selector).prevUntil(stop,filter)
Parameters
This method accepts the following optional parameters −
- stop (optional): A selector expression indicating the element to stop at.
- filter (optional): A string containing a selector expression to filter the results.
Example 1
In the following example, we are using the prevUntil() method to select all the sibling elements between the <div> elements with class "start" and "end" −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $(".target").prevUntil(".start").css("background-color", "yellow"); }); </script> </head> <body> <div class="start">Div element (with class 'start')</div> <p>Paragraph element.</p> <p>Paragraph element.</p> <p>Paragraph element.</p> <div class="target">Div element (with class 'target').</div> </body> </html>
When we execute the above program, selected elements will be highlighted with yellow background color.
Example 2
Here, we are demonstrating the usage of prevUntil() method with "filter" parameter.
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $(".target").prevUntil(".start", ".highlight").css("background-color", "yellow"); }); </script> </head> <body> <div class="start">Div element (with class 'start').</div> <p class="highlight">Paragraph element (with class 'highlight').</p> <p class="highlight">Paragraph element (with class 'highlight').</p> <p>Paragraph element.</p> <div class="target">Div element (with class 'target').</div> <p class="highlight">Paragraph element (with class 'highlight') This element won't be highlighted because it is out side the range of the specified elements.</p> </body> </html>
When we execute the above program, it selects and highlights the elements with class "highlight" in between the <div> elements with class "start" and "target".
Example 3
In the below example, we are returning all elements with class names "one" and "two", that are in between the <div> elements with class "start" and "target" −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $(".target").prevUntil(".start", ".one, .two").css("background-color", "yellow"); }); </script> </head> <body> <div class="start">Div element (with class 'start').</div> <p class="one">Paragraph element (with class 'one').</p> <p class="one">Paragraph element (with class 'one').</p> <p>Paragraph element.</p> <h1>Heading element.</h1> <h1 class="two">Heading element (with class 'two').</h1> <div class="target">Div element (with class 'target').</div> </body> </html>
When we execute the above program, selected elements will be highlighted with yellow background color.